Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial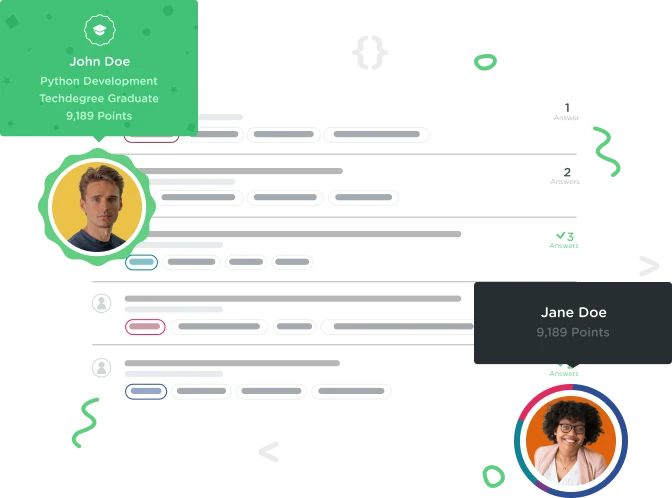

Michael Haupt
Courses Plus Student 521 PointsI keep getting a syntax error for else and I can't figure out why, can anyone point out where my mistake is?
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >=1:
print("There are {} tickets remaining.".format(tickets_remaining))
users_name = input("What is your Name? ")
print("Hello", users_name)
total = input("How many tickets would you like {}? ".format (users_name ))
#expect a ValueError to happen and handle it appropriatly
total == int()
try:
if total > tickets_remaining:
raise ValueError("Sorry there are only {} tickets remaining".format (tickets_remaining))
if total != int():
raise ValueError("Sorry, numbers only")
else:
amount_due = total * TICKET_PRICE
print("Your total will be {}.".format(amount_due))
proceed = input("Do you want to proceed Y/N? ")
if proceed.lower() == "y":
# TODO: Gather credit card
print("SOLD!")
tickets_remaining = tickets_remaining - total
else:
print("Thank you anyway", users_name)
if tickets_remaining == 0:
print("Sold Out")
1 Answer

Zimri Leijen
11,835 PointsIf you want to put code in a comment, use codeblocks.
You can do so like this (the py is for python, you can also use css, js, etc for other languages, which gives it the right color codes, you can also leave it out):
```py
while tickets_remaining >=1: print("There are {} tickets remaining.".format(tickets_remaining)) users_name = input("What is your Name? ") print("Hello", users_name) total = input("How many tickets would you like {}? ".format (users_name )) #expect a ValueError to happen and handle it appropriatly total == int() try: if total > tickets_remaining:
raise ValueError("Sorry there are only {} tickets remaining".format (tickets_remaining)) if total != int(): raise ValueError("Sorry, numbers only") else: amount_due = total * TICKET_PRICE print("Your total will be {}.".format(amount_due)) proceed = input("Do you want to proceed Y/N? ") if proceed.lower() == "y": # TODO: Gather credit card print("SOLD!") tickets_remaining = tickets_remaining - total else: print("Thank you anyway", users_name) if tickets_remaining == 0: print("Sold Out")
```
then it will look something like this (I couldn't be bothered fixing all the indentation etc.):
while tickets_remaining >=1:
print("There are {} tickets remaining.".format(tickets_remaining))
users_name = input("What is your Name? ")
print("Hello", users_name)
total = input("How many tickets would you like {}? ".format (users_name ))
#expect a ValueError to happen and handle it appropriatly
total == int() try: if total > tickets_remaining:
raise ValueError("Sorry there are only {} tickets remaining".format (tickets_remaining)) if total != int(): raise
ValueError("Sorry, numbers only") else: amount_due = total * TICKET_PRICE print("Your total will be
{}.".format(amount_due)) proceed = input("Do you want to proceed Y/N? ") if proceed.lower() == "y": # TODO: Gather
credit card print("SOLD!") tickets_remaining = tickets_remaining - total else: print("Thank you anyway", users_name) if
tickets_remaining == 0: print("Sold Out")
Michael Haupt
Courses Plus Student 521 PointsMichael Haupt
Courses Plus Student 521 PointsI got it formated right, do you have any idea what my issue is?
Zimri Leijen
11,835 PointsZimri Leijen
11,835 PointsAh, you have a Try: and then an Else: on the same level.
I think it should be
try:
andexcept
instead.