Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial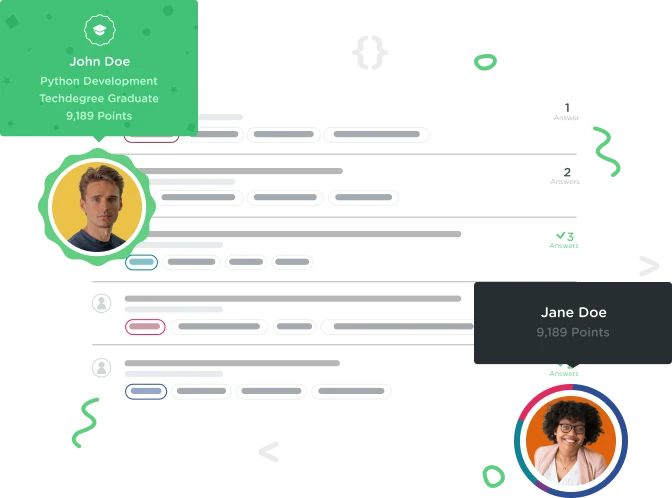
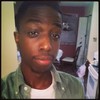
abou93
14,319 PointsI keep getting a syntax error with my code! stuck on the dungeon game
When putting the values of CELLS in my game instead of following like kenneth did I instead chose to format the cells as if it were an x and y formatted graph. with (0, 0) being the middle cell. following an X and Y axis. What this means though is that in my get_moves() function, instead of barring certain x or y values from going left right up or down, i must bar entire cell values from movements. for example if player[0, 1] == -1, 1: moves.remove('LEFT') however the tuples after the equal operator get a syntax error with the comma. Why is this? and is there any other method? here is my code:
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
#player = (x, y)
if player[0, 1] == -1, 1 or -1, 0 or -1, -1:
moves.remove('LEFT')
#If players xy is *(-1, 1)*, (-1, 0), (-1, -1) remove left.
if player[0, 1] == 1, 1 or 1, 0 or 1, -1:
moves.remove('RIGHT')
#if players xy is (1, 1), (1, 0), (1, -1) remove right.
if player[0, 1] == -1, 1 or 0, 1 or 1, 1:
moves.remove('UP')
#if players xy is *(-1, 1)*, (0, 1), (1, 1) remove up.
if player[0, 1] == -1, -1 or 0, -1 or 1, -1:
moves.remove('DOWN')
#if players xy is (-1, -1), (0, -1), (1, -1) remove down.
return moves
3 Answers
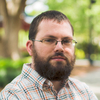
Kenneth Love
Treehouse Guest TeacherOne of the common mistakes in Python, actually.
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
#player = (x, y)
if player == (-1, 1) or (-1, 0) or (-1, -1):
moves.remove('LEFT')
#If players xy is *(-1, 1)*, (-1, 0), (-1, -1) remove left.
if player == (1, 1) or (1, 0) or (1, -1):
moves.remove('RIGHT')
#if players xy is (1, 1), (1, 0), (1, -1) remove right.
if player == (-1, 1) or (0, 1) or (1, 1):
moves.remove('UP')
#if players xy is *(-1, 1)*, (0, 1), (1, 1) remove up.
if player == (-1, -1) or (0, -1) or (1, -1):
moves.remove('DOWN')
#if players xy is (-1, -1), (0, -1), (1, -1) remove down.
You're assuming the left side of the ==
persists. You should have
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
#player = (x, y)
if player == (-1, 1) or player == (-1, 0) or player == (-1, -1):
moves.remove('LEFT')
Or, more easily:
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
#player = (x, y)
if player in [(-1, 1), (-1, 0), (-1, -1)]:
moves.remove('LEFT')
Or even simpler:
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
#player = (x, y)
if player[0] == -1:
moves.remove('LEFT')
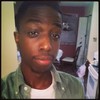
abou93
14,319 Pointshaha yeah i figured it out last night after many attempts. but i didn't realize there were much easier ways to format that. thanks!
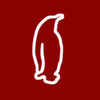
Paxton Butler
6,338 PointsI think that since player is always equal to a tuple (x, y), you can't have the [] after it.
Instead you could say:
if player == 1,0
however, I think that you may be getting a syntax error because you need to compare the player tuple to each move that does not work.
For example to remove Left you would need this entire line of code in the if statement:
if player == (-1,1) or player == (-1,0) or player == (-1,-1):
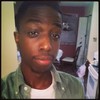
abou93
14,319 Pointsi see my mistake :) lol even after you explained it i still tried for hours trying different things. in if statements, i had if player == only once. i had to declare or player == to each tuple. just like you said. brain fart, but its late thank you very much though!
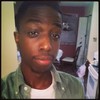
abou93
14,319 Pointsah see I tried it that way as well thinking that would be the case and although the game did start running, i ran into a problem where any input I entered from the list of moves would return *Walls are hard stop running into them" meaning that any move i enter even from the list of moves keeps being a wrong move. I believe its something wrong with the value of moves but i can't seem to pinpoint the problem. again here's my full code:
import random
CELLS = [(-1, 1), (0, 1), (1, 1),
(-1, 0), (0, 0), (1, 0),
(-1, -1), (0, -1), (1, -1)]
def get_locations():
monster = random.choice(CELLS)
door = random.choice(CELLS)
start = random.choice(CELLS)
if monster == door or monster == start or door == start:
return get_locations()
return monster, door, start
def move_player(player, move):
#player = (x, y)
x, y = player
if move == 'LEFT':
x -= 1
elif move == 'RIGHT':
X += 1
elif move == 'UP':
y += 1
elif move == 'DOWN':
y -= 1
return x, y
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
#player = (x, y)
if player == (-1, 1) or (-1, 0) or (-1, -1):
moves.remove('LEFT')
#If players xy is *(-1, 1)*, (-1, 0), (-1, -1) remove left.
if player == (1, 1) or (1, 0) or (1, -1):
moves.remove('RIGHT')
#if players xy is (1, 1), (1, 0), (1, -1) remove right.
if player == (-1, 1) or (0, 1) or (1, 1):
moves.remove('UP')
#if players xy is *(-1, 1)*, (0, 1), (1, 1) remove up.
if player == (-1, -1) or (0, -1) or (1, -1):
moves.remove('DOWN')
#if players xy is (-1, -1), (0, -1), (1, -1) remove down.
return moves
monster, door, player = get_locations()
while True:
moves = get_moves(player)
print("Welcome to the dungeon!")
print("You're currently in room {}".format(player))
print("You can move {}".format(moves))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == "QUIT":
break
if move in moves:
player = move_player(player, move)
else:
print("** Walls are hard, stop walking into them! **")
continue
if player == door:
print("You escaped!")
break
elif player == monster:
print("You were eaten by the grue!")
break
abou93
14,319 Pointsabou93
14,319 PointsHere are my CELLS coordinates:
I have also tried:
if x, y in player == -1, 1:
but with no avail the same syntax error with the commas occur