Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial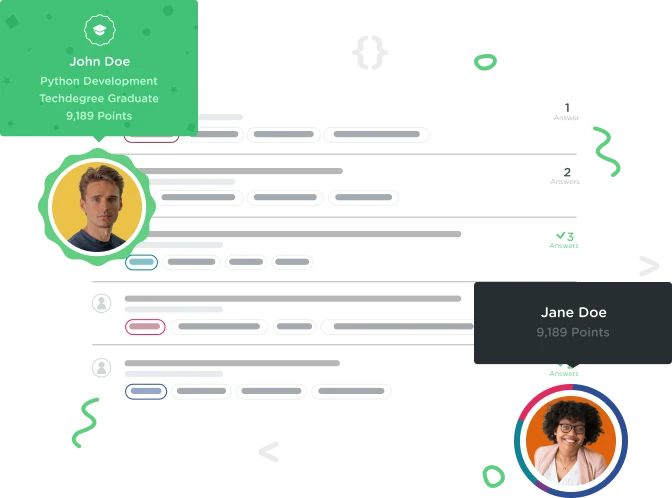

David P
Courses Plus Student 1,162 Pointsi keep getting a syntax error....what am I doing wrong?
This challenge is similar to an earlier one. Remember, though, I want you to practice! You'll probably want to use try and except on this one. You might have to not use the else block, though. Write a function named squared that takes a single argument. If the argument can be converted into an integer, convert it and return the square of the number (num ** 2 or num * num). If the argument cannot be turned into an integer (maybe it's a string of non-numbers?), return the argument multiplied by its length. Look in the file for examples.
def squared(5):
try:
int(5)
except:
return int(5) ** 2
else:
return 5 * len(5)
1 Answer

Krahr Chaudhury
Courses Plus Student 139 PointsThere's a LOT of mistakes in this code - I suggest you go through the videos/tutorials again.
When defining a function you should leave the argument to be a variable - like x. Not a constant value - like 5.
After defining the function, THEN you call it passing a constant or a variable that has a defined value as the variable.
Inside 'try', you should be converting the input variable (in the code below, I've used x) to an integer. Inside 'except', you include a fallback code for when int() doesn't work on the input (i.e. when the input is a string that doesn't read a number). So don't call int() again! We know it doesn't work. Instead call len() on the input to get its string length and return the square of that. Inside 'else' (which is where the program goes when the bit in try succeeds), you return the square of x. No need to call len().
def squared(x):
try:
x = int(x) # Ensure x is converted to an integer.
except:
return len(x) ** 2 # if x is a string that can't be translated to a number, then square its length
else:
return x * x # otherwise square the integer
print squared(5) # x=5, int(5) = 5, 5*5 = 25
print squared("3") #x="3", int("3") = 3, 3*3 = 9
print squared("boo") #x="boo", int("boo") doesn't work! so do len("boo") = 3, 3**2 = 9
Hunter Gass
4,878 PointsHunter Gass
4,878 Pointskoha your code doesn't work either