Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial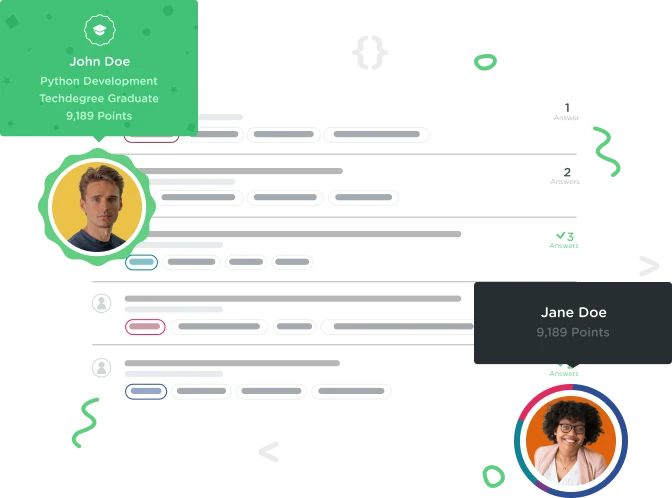

Phoenix S.
2,456 PointsI keep getting an error even though my code is the exact same
Here's my Code for my files from the video. It keeps failing with the error message:
Karaoke.java:15: error: unreported exception IOException; must be caught or declared to be thrown
KaraokeMachine machine = new KaraokeMachine(songBook);
^
1 error
Karaoke.java
import com.teamtreehouse.KaraokeMachine;
import com.teamtreehouse.model.Song;
import com.teamtreehouse.model.SongBook;
public class Karaoke {
public static void main(String[] args) {
// Song song = new Song(
// "Michael Jackson",
// "Beat It",
// "https://www.youtube.com/watch?v=SaEC9i9QOvk");
SongBook songBook = new SongBook();
// System.out.printf("Adding %s %n", song);
// songBook.addSong(song);
// System.out.printf("There are %d song. %n", songBook.getSongCount());
KaraokeMachine machine = new KaraokeMachine(songBook);
machine.run();
}
}
KaraokeMachine.java
package com.teamtreehouse;
import com.teamtreehouse.model.Song;
import com.teamtreehouse.model.SongBook;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map;
public class KaraokeMachine {
private SongBook mSongBook;
private BufferedReader mReader;
private Map<String, String> mMenu;
public KaraokeMachine(SongBook songBook) throws IOException {
mSongBook = songBook;
mReader = new BufferedReader(new InputStreamReader(System.in));
mMenu = new HashMap<String, String>();
mMenu.put("add", "Add a new sont to the song book");
mMenu.put("quit", "Give up? Exit the program");
}
private String promptAction() throws IOException {
System.out.printf("There are %d songs available. Your options are %n", mSongBook.getSongCount());
for (Map.Entry<String, String> option : mMenu.entrySet()) {
System.out.printf("%s - %s %n", option.getKey(), option.getValue());
}
System.out.print("What do you want to do: ");
String choice = mReader.readLine();
return choice.trim().toLowerCase();
}
public void run() {
String choice = "";
do {
try {
choice = promptAction();
switch (choice) {
case "add":
//TODO: Add a new song
Song song = promptNewSong();
mSongBook.addSong(song);
System.out.printf("%s added! %n%n", song);
break;
case "quit":
System.out.println("Thanks for playing!");
break;
default:
System.out.printf("Unknown choice: '%s'. Try again. %n%n%n", choice);
}
}
catch(IOException ioe) {
System.out.println("There was a problem with your input");
ioe.printStackTrace();
}
}
while(!choice.equals("quit"));
}
private Song promptNewSong() throws IOException {
System.out.print("Enter the artist's name: ");
String artist = mReader.readLine();
System.out.print("Enter the title: ");
String title = mReader.readLine();
System.out.print("Enter the video URL: ");
String videoURL = mReader.readLine();
return new Song(artist, title, videoURL);
}
}
2 Answers

Emmanuel C
10,636 PointsIn the instructors code the KaraokeMachine constructor doesnt throw an IOException. If you want to keep that you have to try catch where you create the object in the main method.

Phoenix S.
2,456 PointsFor whatever reason I initially had it that way and it STILL didn't work but it worked when I removed it just now. Sooo frustrating!! Thanks for your answer! :)