Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial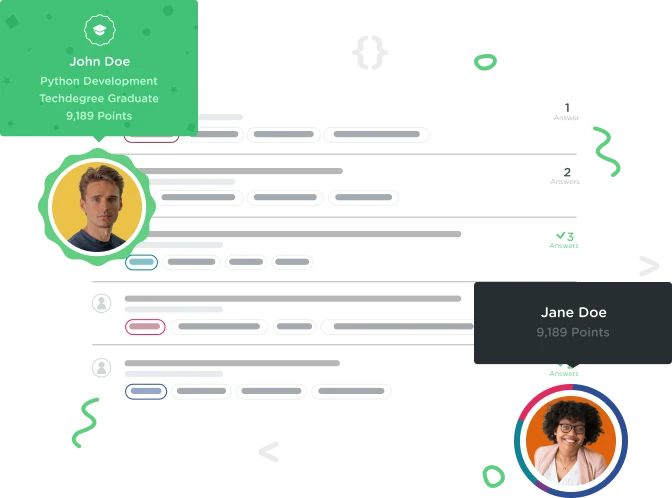
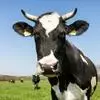
Ava Jones
10,767 PointsI keep getting an error that I am trying to raise help!!
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
print("TICKETS REMAINING: {}".format(tickets_remaining))
username = input("What is your name? ")
num_tickets = int(input("Hey {} how many tickets would you like to buy? ".format(username)))
#expect an ValueError and handle it
try:
num_tickets = int(num_tickets)
except ValueError:
print("Oh No an error! please try again")
else:
total_price = num_tickets * TICKET_PRICE
print("THE TOTAL IS: {}".format(total_price))
buy_tickets = input("Do you want to confirm? YES/NO ")
if buy_tickets .lower() == "yes":
print("SOLD!")
tickets_remaining -= num_tickets
else:
print("Thank you anyways {}!!!!".format(username))
print("ALL SOLD OUT! :(")
1 Answer
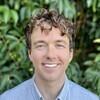
Asher Orr
Python Development Techdegree Graduate 9,410 PointsHi Ava! Try moving your num_tickets = int(input("Hey {} how many tickets would you like to buy? ".format(username)))
inside the try block. Like this:
#expect an ValueError and handle it
try:
num_tickets = int(
input("Hey {} how many tickets would you like to buy? ".format(username)))
num_tickets = int(num_tickets)
except ValueError:
print("Oh No an error! please try again")
else:
total_price = num_tickets * TICKET_PRICE
In the first version of your code, you ask the user how many tickets they want. You added the int(input
, which tells Python to save num_tickets as an integer. Good stuff! The problem is that it's outside the try block.
If someone enters a non-integer for num_tickets, the program crashes because it's outside the try block. Putting it inside that try block tells Python "hey, I think someone might make an error here. Be ready to catch it!"
PS: You should also make this change to your code to avoid the program crashing again:
else:
total_price = num_tickets * TICKET_PRICE
print("THE TOTAL IS: {}".format(total_price))
Let me explain why like this:
#try this:
try:
#ask the user to type how many tickets they want. Make their result an integer, and save it as num tickets.
num_tickets = int(input("Hey {} how many tickets would you like to buy? ".format(username)))
#note: since you already made num_tickets an integer, you don't need this code -> num_tickets = int(num_tickets)
#unless they create a ValueError (by entering a non-integer)
except ValueError:
#print the error message
print("Oh No an error! please try again")
#but if they don't raise an error message
else:
#do this:
total_price = num_tickets * TICKET_PRICE
#total_price just got defined in the try block.
#python won't remember it outside the try block (you'll learn more about this later.)
#so make sure the print statement using total_price is within the try_block.
print("THE TOTAL IS: {}".format(total_price))
#otherwise you'll get a NameError
#keep up the good work!
Ava Jones
10,767 PointsAva Jones
10,767 PointsThank you so much for helping!
Asher Orr
Python Development Techdegree Graduate 9,410 PointsAsher Orr
Python Development Techdegree Graduate 9,410 PointsYou're welcome! Don't forget to mark "best answer," so other people know you've solved your problem!