Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial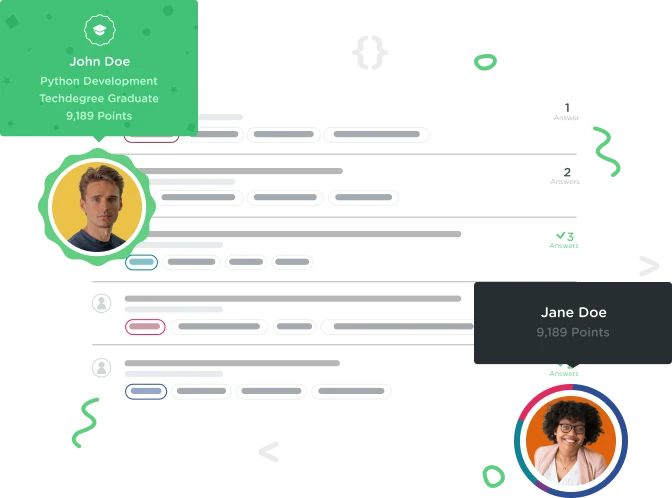
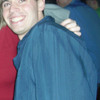
Blake Baxendell
16,344 PointsI keep getting error is not defined.
Here is my console output:
ReferenceError: error is not defined
at getProfile (/Volumes/Passport/Learning/JS/node/lesson_1/app.js:44:34)
at Array.forEach (native)
at Object.<anonymous> (/Volumes/Passport/Learning/JS/node/lesson_1/app.js:51:7)
at Module._compile (module.js:570:32)
at Object.Module._extensions..js (module.js:579:10)
at Module.load (module.js:487:32)
at tryModuleLoad (module.js:446:12)
at Function.Module._load (module.js:438:3)
at Module.runMain (module.js:604:10)
at run (bootstrap_node.js:383:7)
/Volumes/Passport/Learning/JS/node/lesson_1/app.js:39
const message = `There was an error getting the profile for ${username} (${http.STATUS_CODE[response.statusCode]})`;
^
TypeError: Cannot read property '404' of undefined
at ClientRequest.https.get (/Volumes/Passport/Learning/JS/node/lesson_1/app.js:39:98)
at ClientRequest.g (events.js:292:16)
at emitOne (events.js:96:13)
at ClientRequest.emit (events.js:188:7)
at HTTPParser.parserOnIncomingClient [as onIncoming] (_http_client.js:474:21)
at HTTPParser.parserOnHeadersComplete (_http_common.js:99:23)
at TLSSocket.socketOnData (_http_client.js:363:20)
at emitOne (events.js:96:13)
at TLSSocket.emit (events.js:188:7)
at readableAddChunk (_stream_readable.js:176:18)
Here is my JS:
//Problem: We need a simple way to look at a user's badge count and Javascript points
const https = require('https');
//Require http for modules
const http = require('http');
//Print Error Messages
function printError(error) {
console.error(error);
}
const username = "blakebaxendell";
//Solution: Use node.js to connect to treehouse api to get profile information to print out
function printMessage(username, badgeCount, points) {
const message = `${username} has ${badgeCount} total badge(s) and ${points} points in Javascript`;
console.log(message);
}
//Connect to Treehouse API (https://teamtreehouse.com/username.json)
function getProfile(username) {
try {
const request = https.get(`https://teamtreehouse.com/${username}.json`, (response) => {
// Read the data
if(response.statusCode === 200){
let body = '';
response.on('data', (info) => {
body += info.toString();
});
response.on('end', () => {
try{
// Parse the data
const profile = JSON.parse(body);
// Print the data
printMessage(username, profile.badges.length, profile.points.JavaScript);
} catch(error) {
printError(error);
}
});
} else {
const message = `There was an error getting the profile for ${username} (${http.STATUS_CODE[response.statusCode]})`;
const statusCodeError = new Error();
printError(statusCodeError);
}
});
request.on('error', printError(error));
} catch(error) {
printError(error);
}
}
//console.log(process.argv);
const users = process.argv.slice(2);
users.forEach( getProfile);
1 Answer
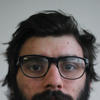
Zack Lee
Courses Plus Student 17,662 Pointsat the line
request.on('error', printError(error));
it should just read "printError" without passing it an error.
you can find the line the error is occurring in the console output
at getProfile (/Volumes/Passport/Learning/JS/node/lesson_1/app.js:44:34)
where it says "app.js:44:34" it means at line 44 at character 34. This isn't always neccessarily the place where the error is actually occurring, but its a good place to start tracking down the errors source.
note later it says
/Volumes/Passport/Learning/JS/node/lesson_1/app.js:39
const message = `There was an error getting the profile for ${username} (${http.STATUS_CODE[response.statusCode]})`;
this could actually be the original source of the error, but because your error catcher had a syntax error the console displayed the error you posted.
fix the first error (line 44) and see how things change. then attempt to fix the second error (line 39) if it still occurs
Blake Baxendell
16,344 PointsBlake Baxendell
16,344 PointsThank you,, it came down to multiple things. It seems he changed a lot of stuff in the code without mentioning it in the video. I eventually had to download his code to see the difference.