Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial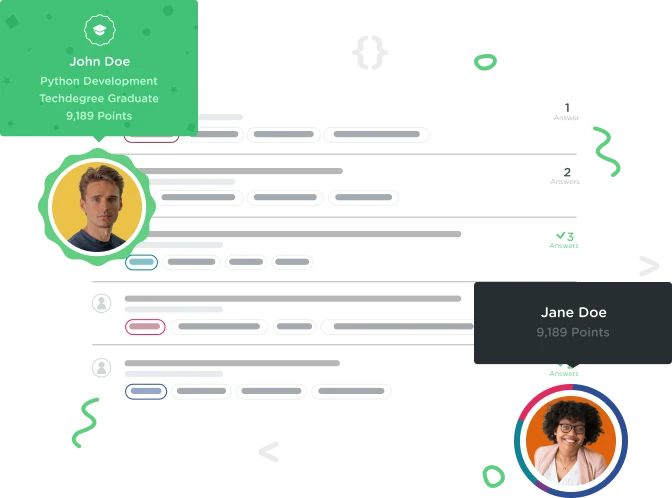

daniel zaragoza
1,843 Pointsi keep getting illegal start of expression error. Help
I'm trying to create a list of words i want to censor but i keep getting the "illegal start of expression " error. Here is the code below.
import java .io.Console;
public class HelloWorld{
public static void main(String []args){
Console console = System.console();
String user = console.readLine(" Enter age");
int age = Integer.parseInt(user);
if(age < 18 ){
console.printf("Sorry you're too young. Exiting!");
System.exit(0);
}
String [] myList ={"stupid", "dummy", "douchebag", "dork", "jerk", "idiot", "nerd"};
String name =;
boolean inValid =;
do{
name = console.readLine("Enter Name");
inValid = (name.equalsIgnoreCase(myList));
if(inValid){
console.printf("Sorry that language is not allowed. Try again. \n\n" );
}
} while(inValid);
}
}
1 Answer
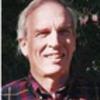
jcorum
71,830 PointsOne problem is that you can't do this:
String name =;
boolean inValid =;
I suggest you do this instead:
String name;
boolean inValid = true;
That is, leave name uninitialized, as you provide a value later. But initialize inValid to true, as you will test and change it to false if the name is invalid.
Next, the compiler doesn't like this:
inValid = (name.equalsIgnoreCase(myList));
because myList is an array, but the equalsIgnoreCase() method takes a String. What you probably intended to do was loop through the array and compare name to each name in the array. But you are not doing that here.