Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial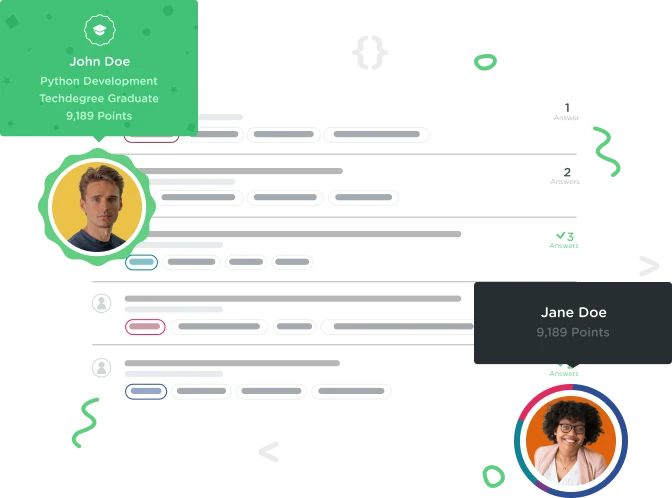
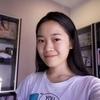
Amber Lim
4,707 PointsI keep getting the number 1 for attempts. Why?
Here's my code. I commented out what I did different from Dave.
var upper = 10000000;
var getRandomNumber = randomNumber(upper);
var guess;
var attempts = 0;
function randomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
while ( guess !== getRandomNumber ) {
guess = getRandomNumber; //Dave used randomNumber(upper) instead.
attempts += 1;
}
document.write("<p>The random number was " + getRandomNumber + "</p>")
document.write("<p>It took " + attempts + " times to guess it right!</p>")
I don't understand how using randomNumber(upper)
over getRandomNumber
in the while loop can produce a different result. Using getRandomNumber
gets me the number 1 for attempts
no matter how many times I refresh it. Once I change my syntax to match Dave's, it worked.
I appreciate any help. Thank you!
3 Answers
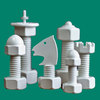
Steven Parker
229,744 PointsYour first guess is always correct, so it only takes one.
When you assign getRandomNumber at the beginning of the program, you put a random number into it. But then later, in the loop, you check that number against itself, so it will always match on the first attempt.
What the original code did was to pick a different random number each time in the loop, and try over and over again until it picked one that matched. If the range is large, that could take a while!

andren
28,558 PointsgetRandomNumber
has a random number generated for it at the start that acts as the solution. By setting the guess
equal to it you are essentially saying guess = solution
it shouldn't be too surprising that you then get it right in one attempt. The while loop is setup to only run when the guess
is not equal to getRandomNumber
so the loop will exit immediately due to your code change.
The randomNumber
function generates a new random number every time it is called, so by using that you are not guaranteed to get the same number that was assigned to getRandomNumber
on the first attempt.
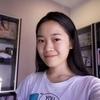
Amber Lim
4,707 PointsThanks for your answer. Helped a ton!
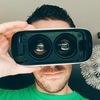
Julien Collet
5,968 PointsAs already said, the getRandomNumber variable contained the integer that was returned by the randomNumber function.
To make your code work as you wrote it, the getRandomNumber variable should contain a function that return the result of the randomNumber function (this don't seems to have much sens though, the Dave's method looks more straightforward).
So this would work :
var upper = 10000000;
var getRandomNumber = function(){return randomNumber(upper);};
var guess;
var attempts = 0;
function randomNumber(upper) {
return Math.floor( Math.random() * upper ) + 1;
}
while ( guess !== getRandomNumber() ) {
guess = getRandomNumber(); //Dave used randomNumber(upper) instead.
attempts += 1;
}
document.write("<p>The random number was " + getRandomNumber() + "</p>")
document.write("<p>It took " + attempts + " times to guess it right!</p>")
Amber Lim
4,707 PointsAmber Lim
4,707 PointsThank you! It makes sense now.