Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial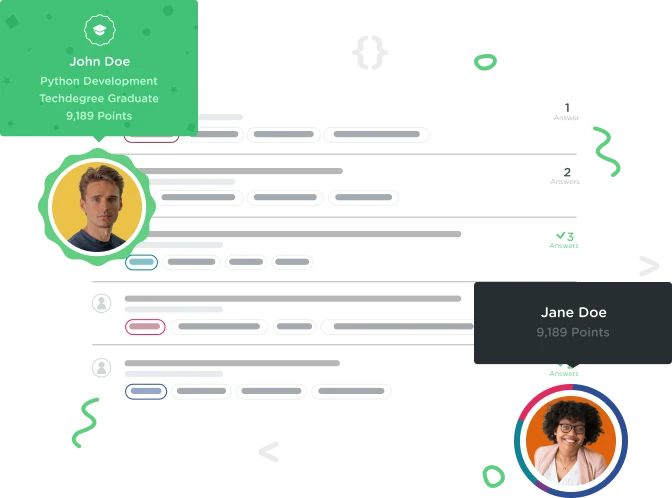

Daniel Fox
10,117 PointsI keep getting this error, and I'm not understanding why? StudentsCode.cs(23,0): error CS1525: Unexpected symbol `{'
I've compared this code to how I structured other If, Else If, and Else statements, and unfortunately, I'm not seeing what I'm missing. Hopefully another set of eyes can help explain this to me. Thanks for the help!!!
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature < 21)
{
System.Console.WriteLine("Too Cold!");
}
else if(temperature == 21)
{
System.Console.WriteLine("Just right.");
}
else if(temperature == 22)
{
System.Console.WriteLine("Just right.");
}
else(temperature > 22)
{
System.Console.WriteLine("Too hot!");
}
2 Answers

andren
28,558 PointsThe problem is that you provide a condition for your else
statement. An else
statement runs automatically if the above if/else if
statements do not run, therefore it does not accept any condition on its own.
You can fix the issue by either changing it into an else if
statement which does accept conditions, or by simply removing the condition all together since its not actually needed. The if/else if
conditions above it already cover less than 21 degrees and 21 to 22 degrees so an else statement would only include values other than those. Like this:
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature < 21)
{
System.Console.WriteLine("Too Cold!");
}
else if(temperature == 21)
{
System.Console.WriteLine("Just right.");
}
else if(temperature == 22)
{
System.Console.WriteLine("Just right.");
}
else
{
System.Console.WriteLine("Too hot!");
}
Also just as a bit of a tip, you don't need two else if
statements to print "Just right." you can accomplish the task with just one by having the condition be <= 22
. That way it will run for 21 but also for 22. Like this:
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature < 21)
{
System.Console.WriteLine("Too Cold!");
}
else if(temperature <= 22)
{
System.Console.WriteLine("Just right.");
}
else
{
System.Console.WriteLine("Too hot!");
}

Daniel Fox
10,117 PointsThank you so much for the help, and for explaining it so clearly!