Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial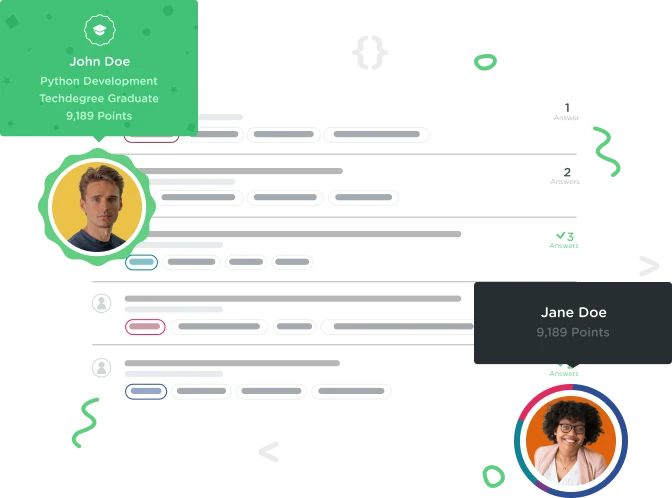

zakaria arr
6,347 PointsI keep getting this error StudentDoesNotExist and OperationalError Everything else looks fine. What wrong with my code?
from peewee import *
db = SqliteDatabase('students.db')
class Student(Model):
username = CharField(max_length=255, unique=True)
points = IntegerField(default=0)
class Meta:
database = db
students = [
{'username': 'bigman',
'points': 3324},
{'username': 'knucklehead',
'points': 3343},
{'username': 'strongman',
'points': 33434},
{'username': 'hench',
'points': 34524},
]
def top_student():
student = Student.select().order_by(Student.points.desc()).get()
return student
def add_students():
for student in students:
try:
Student.create(username=student['username'],
points=student['points'])
except IntegrityError:
student_record = Student.get(username=student['points'])
student_record.save()
if __name__ == '__main__':
db.connect()
db.create_tables([Student], safe=True)
add_students()
print('our top student is: {0.username}.'.format(top_student()))
in workspaces I get
File "/usr/local/pyenv/versions/3.4.1/lib/python3.4
/site-packages/peewee.py", line 2439, in get
% self.sql())
peewee.StudentDoesNotExist: Instance matching query d
oes not exist:
SQL: SELECT "t1"."id", "t1"."username", "t1"."points"
FROM "student" AS t1 WHERE ("t1"."username" = ?)
PARAMS: ['3324']
and in idle I get it somehow works can someone please explain why?? on idle I get
our top student is: hench.
3 Answers
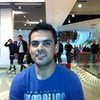
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi Zakaria I created a function that checks to see if each user exists before adding it to the database. see below.
def add_students():
for student in students:
uname = student['username']
upoints = student['points']
try:
if student_exists(uname,upoints) < 1:
Student.create(username=uname,
points=upoints)
except IntegrityError:
print("Error adding students")
def student_exists(uname,upoints):
student = Student.select().where(Student.username==uname,Student.points==upoints)
return student.count()
I ran the code over and over with no errors. I also added new students to the dictionary with higher points and it all seems to work fine now.
hope this helps.

zakaria arr
6,347 PointsKenneth Love can you help me
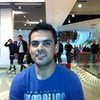
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi Zakaria
the line that prints out the top student, what is {0.username}?? I changed your code slightly, in the function top_student, i returned the student.username rather than just student and also changed the print statement, i then ran your code with no errors. see below
from peewee import *
db = SqliteDatabase('students.db')
class Student(Model):
username = CharField(max_length=255, unique=True)
points = IntegerField(default=0)
class Meta:
database = db
students = [
{'username': 'bigman',
'points': 3324},
{'username': 'knucklehead',
'points': 3343},
{'username': 'strongman',
'points': 33434},
{'username': 'hench',
'points': 34524},
]
def top_student():
student = Student.select().order_by(Student.points.desc()).get()
# changed the return statement from student to student.username
return student.username
def add_students():
for student in students:
try:
Student.create(username=student['username'],
points=student['points'])
except IntegrityError:
student_record = Student.get(username=student['points'])
student_record.save()
if __name__ == '__main__':
db.connect()
db.create_tables([Student],safe=True)
add_students()
# i changed the {0.username} to just the placeholder
print('our top student is: {}.'.format(top_student()))
hope this helps

zakaria arr
6,347 PointsThank you Andreas My apologies I didn't define the error very well and I only recently realised what was causing the problem. The error is being caused due to an IntegrityError because when I run the script the first time it works because all of the usernames and points are unique. However, once the database saves these into a table I will get an error if I run the program. For the meanwhile I have been able to fix this integrity error by using this piece of code that I have posted below. If you have a better way of dealing with this error I would really appreciate it.
And Thank You again

zakaria arr
6,347 Pointsdef add_students():
for student in students:
try:
Student.create(username=student['username'],
points=student['points'])
except IntegrityError:
#I am dealing with the IntegrityError by
#Telling the user that student already exists
print('student already exists')

zakaria arr
6,347 Pointsrunning this code the first time gives
treehouse:~/workspace$ python students.py
our top student is: hench.
and the second time gives
treehouse:~/workspace$ python students.py
student already exists
student already exists
student already exists
student already exists
our top student is: hench.
zakaria arr
6,347 Pointszakaria arr
6,347 PointsWow thanks pal its works perfectly everytime.