Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial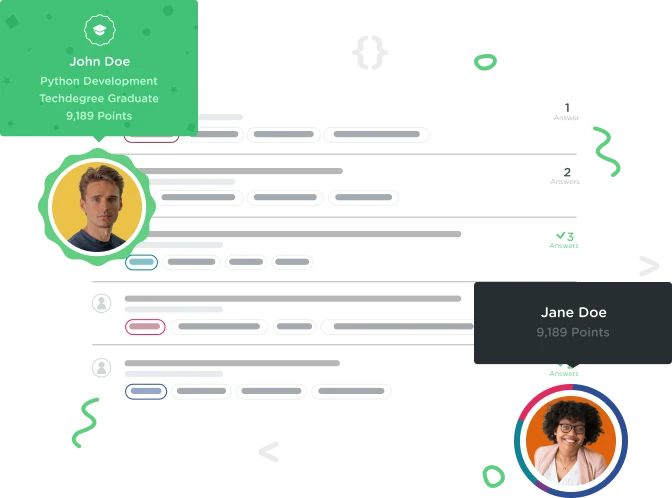

jaap abrahams
9,551 PointsI keep getting "Your setter method is returning the wrong value for the major property." in the setter challenge.
I keep getting "Your setter method is returning the wrong value for the major property." in the setter challenge.
The proper value for the major property is shown upon initializing the student instance (var student = new Student(3.9, 90); -> "Junior"
or
var student = new Student(3.9, 30); -> "None"
).
But if I do this: student.major = 20;
nothing gets updated.
What am I doing wrong?
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
get major() {
return this._major;
}
set major(major) {
this._major = major;
if ( this.level === 'Freshman' || this.level === 'Sophomore' ) {
this._major = 'None';
}
else { this._major = this.level }
console.log(`setter called: ${this.level} - ${this._major}`);
}
}
var student = new Student(3.9, 91);
student.major = 20;
console.log(student.major);
Thanks for some help!
Update:
I adjusted the code and now I get all the proper values in workspace but the challenge checker still returns the error mentioned above. This is the new code (I adjusted the get level
method):
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.major > 90 || this.credits > 90 ) {
return 'Senior';
} else if (this.major > 60 || this.credits > 60 ) {
return 'Junior';
} else if (this.major > 30 || this.credits > 30 ) {
return 'Sophomore';
} else if (this.major < 31 || this.credits < 31 ){
return 'Freshman';
}
}
get major() {
return this._major;
}
set major(major) {
this._major = major;
if ( this.level === 'Freshman' || this.level === 'Sophomore' ) {
this._major = 'None';
}
else { this._major = this.level }
}
}
var student = new Student(3.9, 30);
student.major = 29;
console.log(student.major);
student.major = 31;
console.log(student.major);
student.major = 61;
console.log(student.major);
student.major = 91;
console.log(student.major);
What else can I do? This is getting frustrating : (.
2 Answers
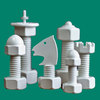
Steven Parker
231,275 PointsThe "level" getter was correct the first time. It has no need to reference "major", which in actual use is likely to be an unrelated string (including "None
"!) instead of a number value.
The "major" setter is using the wrong source to set the backing variable when the student is Junior or Senior. Instead of "this.level
", it should set it using the "major
" parameter that was passed in. If you keep the first line that presets the backing variable, you can eliminate the "else" completely. Otherwise, you could move that line into the "else" block.

jaap abrahams
9,551 PointsThank you! That was the solution. Now I look at the code I am amazed how incredibly simple the problem and its solution are. I will wear my 'shame on me' hat for the rest of the day.
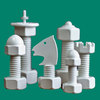
Steven Parker
231,275 PointsThere's no shame in mistakes, they are the best teachers!
Happy coding!