Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial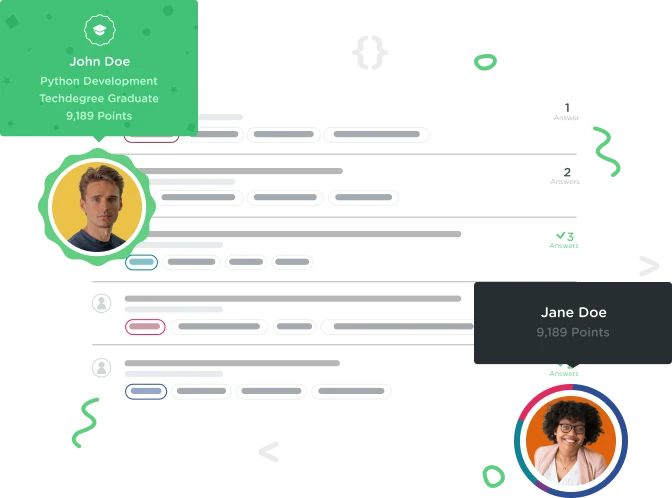

Andrei Oprescu
9,547 PointsI know I am dong something wrong. can someone help me?
I am at the last task of the objects course, and I have a question like this:
And, finally, if I have doubles, I want to reroll the hand. Add a classmethod to CapitalismHand named reroll that returns a new instance of the class, effectively rerolling the hand.
I have rewatched the video various times but I couldn't figure it out.
My code for that challenge is at the bottom of the question.
Can someone tell me how to fix this?
Thanks!
from dice import D6
class Hand(list):
def __init__(self, size=2, die_class=D6, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
@property
def doubles(self):
if self[0] == self[1]:
return True
return False
@classmethod
def reroll(cls):
if self.doubles == True:
return cls(Hand().__init__())
2 Answers
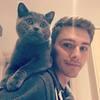
Oliver Small
12,578 PointsHey, good effort! Remember self is not an argument of a @classmethod so therefore you cant use it. Instead you can use the cls argument. You only need to return the class again to roll again. Also as a little tip, to check if something is true you can just do - if self.doubles:
@classmethod
def reroll(cls):
if cls.doubles:
return cls()

Andrei Oprescu
9,547 PointsI have tried your code and your advise, and it gave 'Didn't get a new hand'
Can you please suggest what't wrong?

prateekparekh
12,895 PointsCan you post your code?