Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial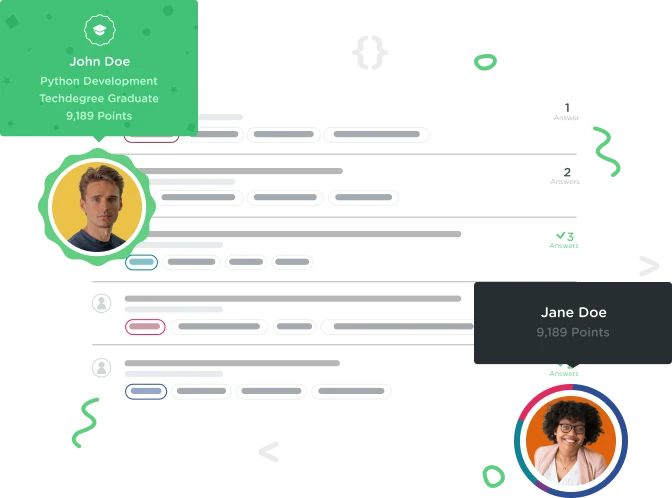
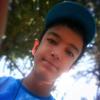
Furkan Demirkan
7,926 PointsI know what I have to do, but am doing it right?? Defaulting Parameters Challenge...
Here's my code. I am clueless on whether or not I can define addItem twice with different parameters and if I'm even doing it right.. Im at the point where my brain freezes and I just stare at my code for hours :D Please help! Thanks in advance!
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
addItem(item, quantity);
}
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
public void addItem(Product item){
addItem(item, 1);
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}

Phillip Hurst
4,204 PointsI don't understand why you have a ", 1" in the addItem line when the method is only passed in 1 attribute of "Product item"?
public void addItem(Product item) {
addItem(item, 1);
}
I think you are supposed to have a print line as well?
public void addItem(Product item) {
System.out.printf("Added 1 of %s to the cart %n", item);
addItem(item);
}
I believe this is what I tried at first for this challenge and think it would not work for some reason? Ended up looking to the forums.
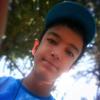
Furkan Demirkan
7,926 PointsPhillip,
The challenge asks us to automatically register a int of '1' if an int isn't given in the parameter, take 'cart.addItem(dispenser);' from Example.java into example. So I wrote another addItem method that takes only 'item' as its parameter. Like I said, the challenge wants us to automatically return an int of 1 if no int is given, therefor I wrote:
public void addItem(Product item){ addItem(item, 1); }
so that if there was only an 'item' in the parameters, it would return the item and 1, just what we need. Since the 'new' 'addItem' has both 'item' and 'int' in its parameters [addItem(Product item, int)], it goes to the other addItem method:, which returns the item and quantity(1):
public void addItem(Product item, int quantity) { System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
Also regarding your code, you don't need the printf, that would just print out that you are returning int 1, but the code actually isn't doing anything. So all in all, your ShoppingCart.java should look something like this:
public class ShoppingCart {
public void addItem(Product item, int quantity) { System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName()); }
public void addItem(Product item) { addItem(item, 1); } }
Sorry for making things complicated, I wouldn't make a good teacher :D If you have any questions, I'm here pretty much all day.
Cheers
1 Answer

Ron Fisher
5,752 PointsFurkan,
Don't be upset at yourself. We all make mistakes. Those who learn from theirs tend not to make them again. That is true learning. Keep up the great work and continue on your trek.
Ron
Furkan Demirkan
7,926 PointsFurkan Demirkan
7,926 PointsEDIT: Im soo mad that I couldnt see it before. I dont want to give it away for others attemting to solve this, but I made a silly mistake here::
public void addItem(Product item, int quantity) { System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName()); addItem(item, quantity); }
Im so frustrated with myself :/