Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial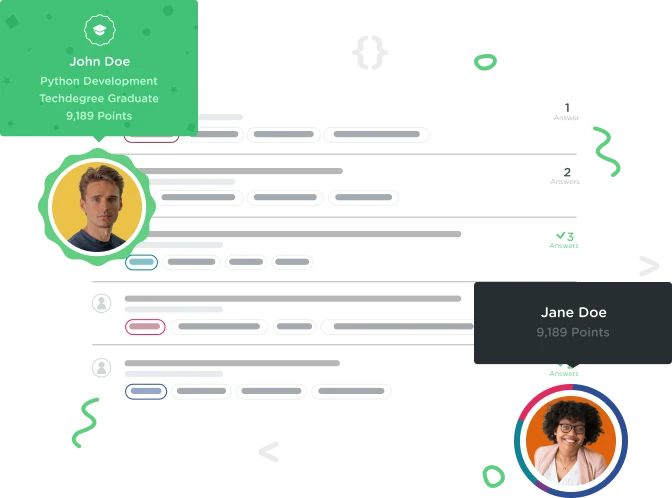

Joe Hobbs
15,882 PointsI literally have no idea on how to do this, help?
Been on this a while and im really confused
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public List<int> GetPowersOf2(int value)
{
return powers[value];
}
}
}
1 Answer
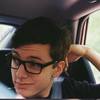
Zachary Steiger
21,391 PointsYou were off to a good start! It's confusing at first but once you break it up it becomes a bit easier to understand. You set up the method correctly, by both declaring the return type to be a List<int>, and setting the correct parameters on GetPowersOf2, all you need to do now is set up the list to return, and then perform the Math.Pow operation.
Because we're using both List<T> and Math classes, you'll have to add the correct using directives up top to start,
using System;
using System.Collections.Generic;
Next, inside your method, instantiate a new variable called returnList so we can return the list of integers to the method
public static List<int> GetPowersOf2(int input)
{
var returnList = new List<int>();
Then create a for loop to populate the list! Setting up the for loop is pretty straightforward, but you have to remember to set your limit to be less than or equal to input otherwise your list will be one integer short (i < input +1 also works). The return block is the tricky part. We have to use the Add method on our new returnList variable to add our integers to the list, and inside the Add method we can directly call Math.Pow, which takes two parameters. The challenge wants every integer to be raised to the value of 2, making our first parameter the number 2! The next parameter is i from our for loop, which is every number up to our users input raised to the power of 2. However Math.Pow only takes the type double, so you'll want to use type-casting and change the return type to int by placing (int) just before Math.Pow()
for (int i = 0; i <= input; i++)
{
returnList.Add((int)Math.Pow(2, i));
}
Next, return the returnList variable back to the GetPowersOf2 method and you're done!
return returnList;
}

Joe Hobbs
15,882 PointsThankyou so much, I also noticed i kept forgetting to made the method a static one so it wasnt allowing me to create an instance of List<int> which then made me think i was doing everything wrong haha, but thanks again!
Shane Robinson
7,324 PointsShane Robinson
7,324 PointsDeleted my potentially unhelpful responses - hopefully somebody can help you out in a timely fashion. :)