Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial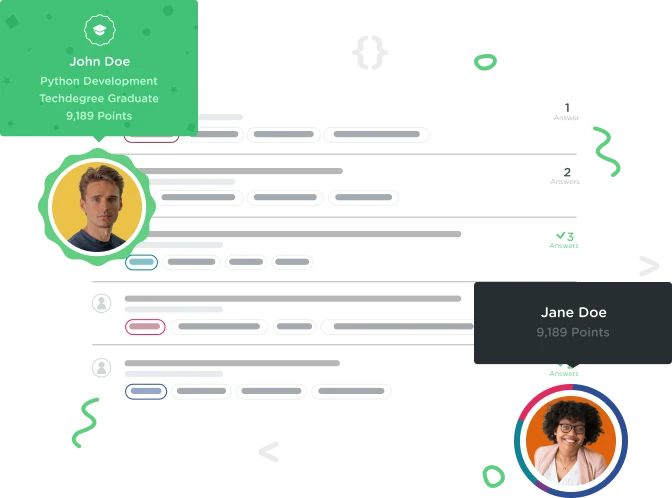
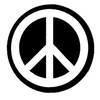
john larson
16,594 PointsI looked in the MDN, stack overflow, and w3schools and I couldn't find how to sort an object within an array...
I would like to print the names alphabetically from these objects nested within an array. Here is the array/object
var students = [
{
name: "Dionne",
position: "Matriarch",
title: "Derblin Prime",
subjugation: "Supreme"
},
{
name: "Soup Can",
position: "Daughter1",
title: "Derblin Squared",
subjugation: "Lateral"
},
{
name: "Sageling",
position: "Daughter2",
title: "Derblin Cubed",
subjugation: "Lateral"
},
{
name: "Soup Ladel",
position: "Patriarch",
title: "Derblin Extraneous",
subjugation: "underling"
}
];
here is the code to print the names
var i;
var names = "";
var message = "";
names += "<p>Student names: </p>";
for(i = 0; i < students.length; i += 1){
names += "<li>" + students[i].name + "</li> ";
print(names);
}
it prints the names fine but it would make sense for them to be in alphabetical order...if that's possible
5 Answers

Qasim Hafeez
12,731 Pointsjohn larson,
This is how I got it to work
function compare(s1, s2){
if(s1.name > s2.name){
return 1;
} else if (s1.name < s2.name){
return -1;
} else{
return 0;
}
}
students.sort(compare);
var i;
var names = "";
var message = "";
names += "<p>Student names: </p>";
for(i = 0; i < students.length; i += 1){
names += "<li>" + students[i].name + "</li> \n";
}
console.log(names);
I'm not clear on how exactly the compare function works in this case but, it lists the names alphabetically for me. Also, you say it printed out the names for you but when I ran your code the print window popped up. I used console.log to print the names to the console. You'll notice I put the 'console.log(names)' on the outside of the loop, otherwise it prints out four lists of names, with the only complete one being the last one.

srikarvedantam
8,369 PointsYou can use the "sort(...)" method available on arrays to sort its items. Further, you need to tell the sort(...) method how to "compare" two items. Here's how you can sort the "students" collection by student's name property:
function compare(s1, s2) {
if (s1.name < s2.name)
return -1;
if (s1.name > s2.name)
return 1;
return 0;
}
students.sort(compare);
Just add the above code before you print the names, so that sorted student names will get printed out.
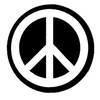
john larson
16,594 PointsHi Srikar, sort() is the first thing I thought to try. I couldn't (and still can't) get it to work. Where would I pass the names, before the loop? after? then there's the issue that the names are actually in an object nested within an array. I don't see how to make this function work. Any additional insight would be greatly appreciated. Thank you

srikarvedantam
8,369 Pointssort(...) is a built-in method; it is expected to run faster than a typical implementation. If it's speed is not satisfactory, a custom sort routine needs to be implemented and "Sorting" techniques, one of the oldest and long studied computing problem, warrants a different course altogether.

Qasim Hafeez
12,731 PointsHere's a stackoverflow link that answers how it works:
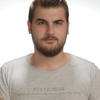
Erdem Meral
25,022 PointsAlso another problem is the speed. I dont think that the solution above is fast enough.
Hope Dave can help us :)
john larson
16,594 Pointsjohn larson
16,594 PointsThanks Quasim, That weird print thing that pops up sometimes, don't know what up with that. I thought it was some glitch on my pc but...it happened to you too. I got it to print to the page though, thanks. I would ask you to explain some of it to me but...sounds like it a bit of a mystery to you too. Peace my friend :D