Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial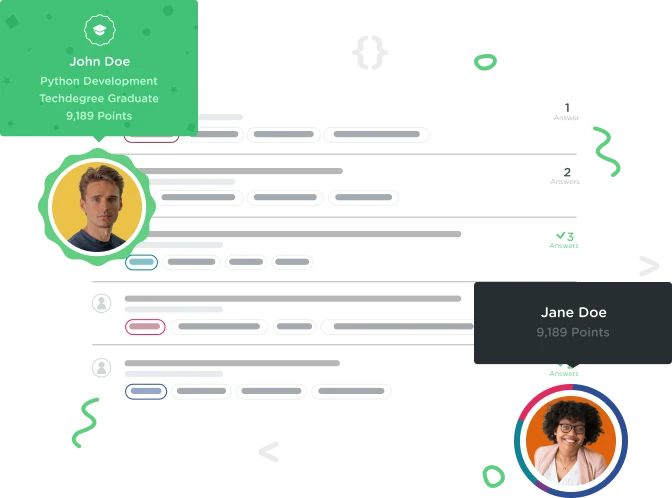

Luis Santos
3,566 PointsI lost myself here, again....
It's asking me for a bool value, I am having immense trouble attempting to figure this out. Any help would be appreciated, thank you.
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
bool EatFly (int distanceToFly) {
return EatFly;
}
}
}
}
3 Answers

Michael Liebegott
2,852 PointsLuis, it can be a bit confusing during the challenges, but I believe Jeremy explains it well enough in his videos.
To elaborate on Mikkel's answer:
Your "bool EatFly" method needs to be public unless otherwise specified or else it defaults to private. You've also stated that the method requires a "distanceToFly" int parameter without providing one between the brackets { }. You need to test whether or not the distance to the fly (distanceToFly) is less than or equal to the length of the frog's tongue (TongueLength) and return a bool value. To do this, you must use an if/else statement, which in plain English would be:
If the length of the frog's tongue (TongueLength) is greater than or equal to the distance to the fly (distanceToFly), the frog can eat the fly (EatFly = true). Or else the frog cannot eat the fly (EatFly = false).
You can do this in two ways. You can either declare a variable inside of the method and use only one return statement, or you can do what Mikkel did and use two return statements. I'm still new to this as well, so if anyone has any criticisms of my explanation, let me know. If you need more help Luis, I'll try my best.

Mikkel Rasmussen
31,772 PointsI will suggest you to watch some of the videos again because it doesn't look like you fully understood them.
But here is the answer:
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public bool EatFly(int distanceToFly) {
if(TongueLength >= distanceToFly) {
return true;
} else {
return false;
}
}

Luis Santos
3,566 PointsI understand the material, but I get lost when the challenges come up. I think these instructors need a better way of explaining the material.

Sean Cornwell
6,042 PointsIt took me a couple tries as well to figure it out, but you don't need to do an if/else statement for this.
First, you need to make your method public or it will default to private. You probably know this as you did it in the preceding method.
Second, you need to add the simple check to see if EatFly is a true or false value. In this case you can simply set EatFly equal to distanceToFly if less than or equal to TongueLength. This simple equation will return a true value if the distanceToFly is less than or equal to the TongueLength of the frog. It will also return a false value if the equation is false.
It really is exactly what Jeremy did in the video, just a much simpler equation with different names and variables.
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public bool EatFly(int distanceToFly)
{
bool EatFly = distanceToFly <= TongueLength;
return EatFly;
}
}
}