Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial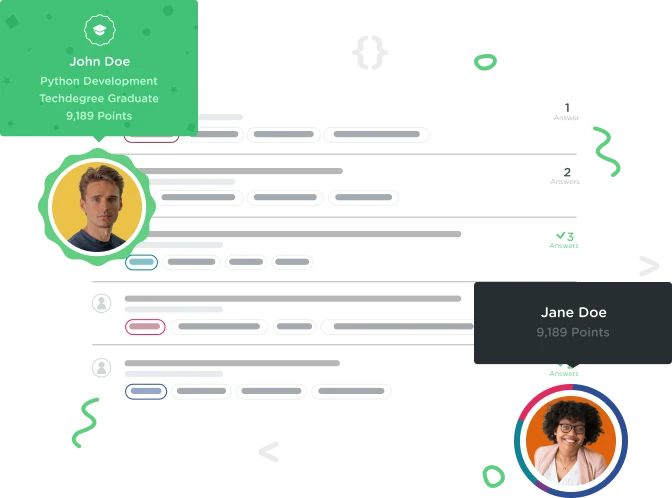
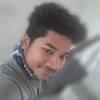
Ashish Mehra
Courses Plus Student 340 PointsI m getting error instead of artist name
function.php
<?php
function generate_Catalog(){
include("connection.php");
try{
$result = $db->query("Select media_id,title,category,img FROM media");
}catch(Exception $e){
echo "Unable to Retreived Result";
echo $e->getMessage();
exit;
}
$catalog = $result->fetchAll(PDO::FETCH_ASSOC);
return $catalog;
}
function generate_single_Catalog($id){
include("connection.php");
try{
$result = $db->prepare(
"Select title,category,img,format,genre,year,publisher,isbn
FROM media
JOIN genres ON media.genre_id = genres.genre_id
LEFT OUTER JOIN books ON media.media_id = books.media_id
WHERE media.media_id = ?"
);
$result->bindParam(1,$id,PDO::PARAM_INT);
$result->execute();
}catch(Exception $e){
echo "Unable to Retreived Result";
echo $e->getMessage();
exit;
}
$item = $result->fetch(PDO::FETCH_ASSOC);
if(empty($item)) return $item;
try{
$result = $db->prepare(
"Select fullname,role
FROM media_people
JOIN people ON media_people.people_id = people.people_id
WHERE media_people.people_id = ?"
);
$result->bindParam(1,$id,PDO::PARAM_INT);
$result->execute();
}catch(Exception $e){
echo "Unable to Retreived Result";
echo $e->getMessage();
exit;
}
while($row = $result->fetch(PDO::FETCH_ASSOC)){
$item[$row["role"]][] = $row["fullname"];
}
return $item;
}
function get_item_html($id,$item) {
$output = "<li><a href='details.php?id="
. $item["media_id"]. "'><img src='"
. $item["img"] . "' alt='"
. $item["title"] . "' />"
. "<p>View Details</p>"
. "</a></li>";
return $output;
}
function array_category($catalog,$category) {
$output = array();
foreach ($catalog as $id => $item) {
if ($category == null OR strtolower($category) == strtolower($item["category"])) {
$sort = $item["title"];
$sort = ltrim($sort,"The ");
$sort = ltrim($sort,"A ");
$sort = ltrim($sort,"An ");
$output[$id] = $sort;
}
}
asort($output);
return array_keys($output);
}
details.php
<?php
include("inc/functions.php");
$catalog = generate_Catalog();
if (isset($_GET["id"])) {
$id = filter_input(INPUT_GET,"id",FILTER_SANITIZE_NUMBER_INT);
$item = generate_single_Catalog($id);
var_dump($item);
}
if (empty($item)) {
header("location:catalog.php");
exit;
}
$pageTitle = $item["title"];
$section = null;
include("inc/header.php"); ?>
<div class="section page">
<div class="wrapper">
<div class="breadcrumbs">
<a href="catalog.php">Full Catalog</a>
> <a href="catalog.php?cat=<?php echo strtolower($item["category"]); ?>">
<?php echo $item["category"]; ?></a>
> <?php echo $item["title"]; ?>
</div>
<div class="media-picture">
<span>
<img src="<?php echo $item["img"]; ?>" alt="<?php echo $item["title"]; ?>" />
</span>
</div>
<div class="media-details">
<h1><?php echo $item["title"]; ?></h1>
<table>
<tr>
<th>Category</th>
<td><?php echo $item["category"]; ?></td>
</tr>
<tr>
<th>Genre</th>
<td><?php echo $item["genre"]; ?></td>
</tr>
<tr>
<th>Format</th>
<td><?php echo $item["format"]; ?></td>
</tr>
<tr>
<th>Year</th>
<td><?php echo $item["year"]; ?></td>
</tr>
<?php if (strtolower($item["category"]) == "books") { ?>
<tr>
<th>Authors</th>
<td><?php echo implode(", ",$item["author"]); ?></td>
</tr>
<tr>
<th>Publisher</th>
<td><?php echo $item["publisher"]; ?></td>
</tr>
<tr>
<th>ISBN</th>
<td><?php echo $item["isbn"]; ?></td>
</tr>
<?php } else if (strtolower($item["category"]) == "movies") { ?>
<tr>
<th>Director</th>
<td><?php echo implode(", ",$item["director"]); ?></td>
</tr>
<tr>
<th>Writers</th>
<td><?php echo implode(", ",$item["writer"]); ?></td>
</tr>
<tr>
<th>Stars</th>
<td><?php echo implode(", ",$item["star"]); ?></td>
</tr>
<?php } else if (strtolower($item["category"]) == "music") { ?>
<tr>
<th>Artist</th>
<td><?php echo implode(", ",$item["artist"]); ?></td>
</tr>
<?php } ?>
</table>
</div>
</div>
</div>
Errors
Notice: Undefined index: artist in C:\xampp\htdocs\pdo\pdo\details.php on line 90
Warning: implode(): Invalid arguments passed in C:\xampp\htdocs\pdo\pdo\details.php on line 90
4 Answers

Andrew Goninon
14,925 PointsIn the second 'try' query in your functions.php it should read:
WHERE media_people.media_id = ?"
Instead of:
WHERE media_people.people_id = ?"

Simon Coates
28,694 Pointsit says there is no value for that index ('artist'). You can do a var_dump() on $item to see what key values are present.Either you're using the wrong key, or $item doesn't have the values you think it does. Unless Alena made a mistake, there should be an artist field for a music item. SO you need to confirm if the problem is not having an artist (can confirm via var_dump on item), or whether there is a fault on the test of whether the item is in the music category.

Nick Dim
12,885 PointsI get the same error. Notice: Undefined index: artist in /home/treehouse/workspace/details.php on line 90 A bit disappointed because there was another error few steps behind.

zacharym
11,154 PointsThe same thing was happening to me, it seems the problem is that the second query is using the same $results object as the first query. Changing $results to $results2, or some other name in the second query made it work for me.