Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial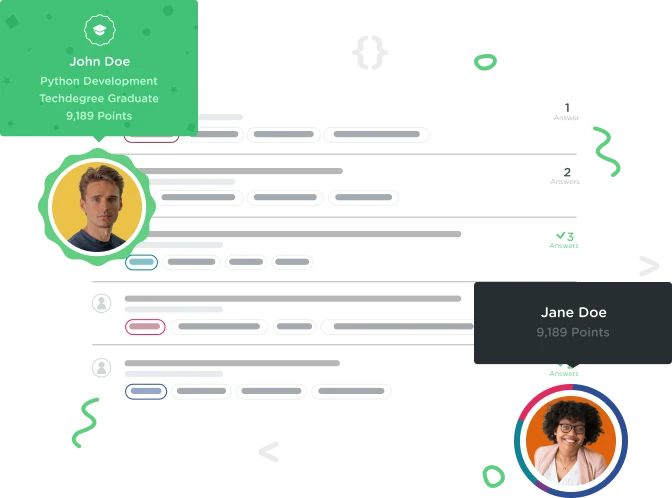
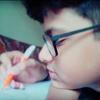
deshmitra mishra
536 PointsI m not understanding what i m doing wrong!
i dont understand what i did wrong here and why it shows that it was expecting "(" at line 6 column 5, i already put the brackets in if statement.
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
public boolean isBatteryEmpty
if (barCount.isBatteryEmpty()) {
System.out.printf("true");
}
if (!barCount.isBatteryEmpty()) {
System.out.printf("false");
}
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
}
1 Answer
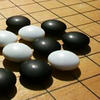
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsThere are several issues:
- your method isBatteryEmpty needs brackets after the method name like this: isBatteryEmpty()
- before you start writing the if statement and the rest of the method, you need curly braces. You have curly braces after the if, but not before. Everything that is inside the method must be inside curly braces
- You're not supposed to print "true" or "false" with printf, but to return the value true or false (without the quotes) like this: 'return true;'
- You're using the method isBatteryEmpty() inside the method itself. There are cases where this is ok, but it doesn't work in this case
You can rewrite it all like this:
public boolean isBatteryEmpty() {
if(barCount == 0) {
return true;
} else {
return false;
}
}
But an even better way to write it is this:
public boolean isBatteryEmpty() {
return barCount == 0;
}
This works because 'barCount == 0' is a boolean expression. It can only be true or false. So if the boolean expression is true, the method returns true, and if it's false, it returns false. The if statement is superfluous.
If you have any follow-up questions, don't hesitate.