Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial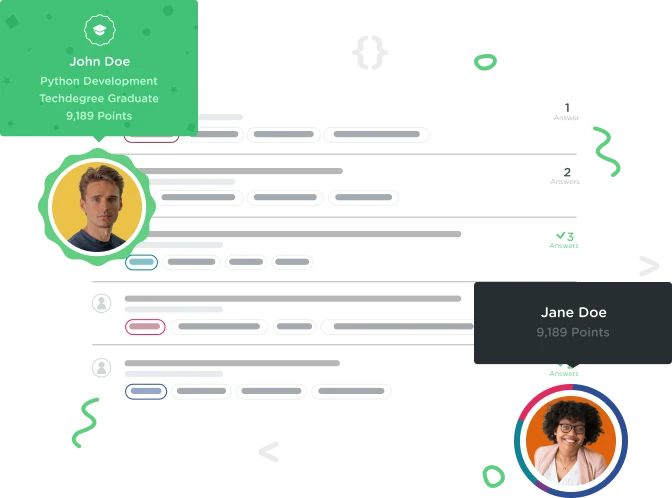
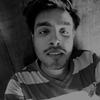
Rohit Sagar
5,938 PointsI m stuck....
I am having hard time to understand parentnode and traverse dom ?
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
4 Answers
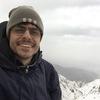
Sam Gord
14,084 PointsHi Rohit, ParentNode gives you the parent element of a selected element with javascript, here in your code you are getting the UL element and assign it to the list variable in this line ( as you already know that ;) )
const list = document.getElementsByTagName('ul')[0];
then you are adding an event listener to the list and telling it to do something JUST if the target of the event (e) had a name of BUTTON , so the event function fires(or runs) only if you click on one of the buttons in the list.
so let me put something in the function in order to make it clear what's gonna happen and tell u what i know about parentNode -->
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
console.log(this.parentNode);
}
});
now if you click on any of the buttons in the list and check the browser's console you see that it shows you the
<section></section>
element . why?
because parentNode gives you the parent of the desired element. but which element? the UL that you assigned the event listener to , not the button that is the target of the event. and the parent of the UL element here is SECTION element .
hope it helped you a little,
happy coding ;)
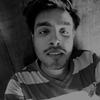
Rohit Sagar
5,938 PointsThanks Again .
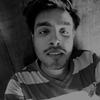
Rohit Sagar
5,938 PointsThis is the main question i m having trouble ... and the code is above i mentioned.......
A delegated click event listener has been attached to the selected ul element, which is stored in the variable list. The handler is targeting each button in the list. When any one of the buttons is clicked, a class of highlight should be added to the paragraph element immediately preceding that button inside the parent list item element. Add the code to create this behavior on line 5.
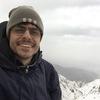
Sam Gord
14,084 Pointsok , now i see the problem .
and here is one simple way of solving it ( it has more than one way but i decided to do it this way so i can use parentNode however! )
lets assume that the highlight class is described like this -->
.highlight{
color: red;
background: green;
}
so we add this class to the paragraphs next to the buttons like this
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let children = e.target.parentNode.childNodes;
//console.log(children);
children[0].classList.add("highlight");
}
});
this it what happens: when we click on a button , we go one level top from that button to its own parent ( e.target.parentNode ) and get any element ( child ) that this parent has (e.target.parentNode.childNodes) and save them to the children array, then we log that array to the console to check out the elements we stored in that array and we see that the paragraph is stored at the first ( 0 index ) position . then we have the freedom to do whatever we want to that element, in this case , adding the highlight class :)
p.s: here is the link to the codepen i created to test the solution , feel free to play around with it if it helps https://codepen.io/simonestic/pen/NLwopy