Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial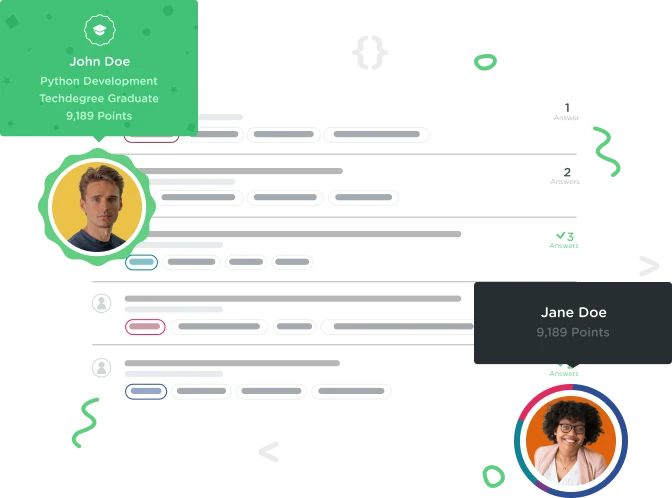

vikk hill
221 PointsI made it really complicated! Is it a good practice to do it like this?
var totalScore = 5;
//First Question
var correctQuestion1 = false;
var question1 = prompt("What is the first Computer language for the a web designer?");
if ( question1.toUpperCase() === "JAVASCRIPT" ) {
correctQuestion1 = true;
} else {
totalScore -= 1;
}
if ( correctQuestion1 ) {
alert("Correct");
} else {
alert("Wrong");
}
//2nd Question
var correctQuestion2 = false;
var question2 = prompt("What is 20+10?");
if ( parseInt(question2) === 30 ) {
correctQuestion2 = true;
} else {
totalScore -= 1;
}
if ( correctQuestion2 ) {
alert("Correct");
} else {
alert("Wrong");
}
//3rd Qestion
var correctQuestion3 = false;
var question3 = prompt("What is the First name of Treehouse's JavaScript Teacher?");
if ( question3.toUpperCase() === "DAVE" ) {
correctQuestion3 = true;
} else {
totalScore -= 1;
}
if ( correctQuestion3 ) {
alert("Correct");
} else {
alert("Wrong");
}
//4th Question
var correctQuestion4 = false;
var question4 = prompt("Is Math Important for JavaScript? Y or N");
if ( question4.toUpperCase() === "YES" || question4.toUpperCase() === "Y" ) {
correctQuestion4 = true;
} else {
totalScore -= 1;
}
if ( correctQuestion4 ) {
alert("Correct");
} else {
alert("Wrong");
}
//5th Question
var correctQuestion5 = false;
var question5 = prompt("What is 20x10?");
if ( parseInt(question5) === 200 ) {
correctQuestion5 = true;
} else {
totalScore -= 1;
}
if ( correctQuestion5 ) {
alert("Correct");
} else {
alert("Wrong");
}
alert("Your total score is " + totalScore);
// Medal for score
if ( totalScore === 5 ) {
document.write("You got Gold medal");
} else if ( totalScore === 3 || totalScore === 4 ) {
document.write("You got Silver Medal");
} else if ( totalScore === 2 || totalScore === 1 ) {
document.write("You got Bronze Medal");
} else {
document.write("Better Luck next Time");
}
2 Answers

James Nakolah
10,542 PointsGood try Vikk. So far, there's absolutely no problem with that. Later in the course, you'll learn why it's not good practice to do it like that. You'll need to utilize the power of loops and functions to improve this code.

KRIS NIKOLAISEN
54,968 PointsYou could simplify your questions as follows:
var question1 = prompt("What is the first Computer language for the a web designer?");
if ( question1.toUpperCase() === "JAVASCRIPT" ) {
alert("Correct");}
else {
alert("Wrong");
totalScore -= 1;
}

vikk hill
221 PointsThank you so much!!