Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial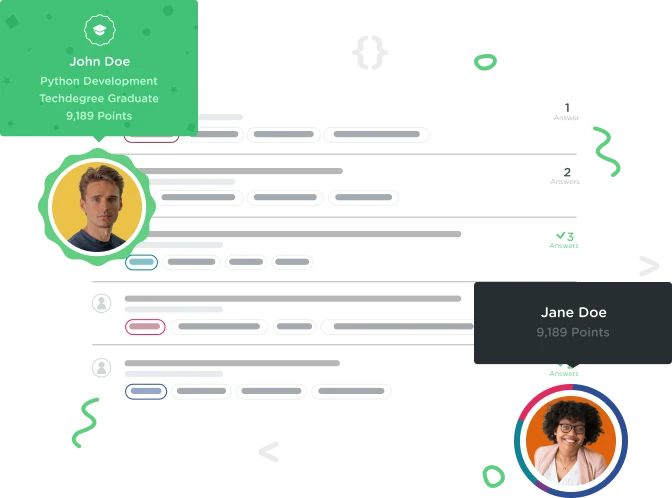

Pitrov Secondary
5,121 PointsI made this game differently, is my code ok?
I made this game, It works fine, but Kenneth made the game differently. is my code OK, or how could I make it better? Here's my code:
import random
CELLS = [(0, 0), (1, 0), (2, 0), (3, 0), (4, 0),
(0, 1), (1, 1), (2, 1), (3, 1), (4, 1),
(0, 2), (1, 2), (2, 2), (3, 2), (4, 2),
(0, 3), (1, 3), (2, 3), (3, 3), (4, 3),
(0, 4), (1, 4), (2, 4), (3, 4), (4, 4),
]
def unpacking_list(list):
string = ''
for item in list:
string += ' {}'.format(item)
return string
def move_player(player_, move):
players_location = [player_[0][0], player_[0][1]]
if move == 'LEFT':
players_location[0] -= 1
if move == 'RIGHT':
players_location[0] += 1
if move == 'UP':
players_location[1] -= 1
if move == 'DOWN':
players_location[1] += 1
return players_location
def get_moves(player_position):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN',]
if player_position[0][1] == 0:
del moves[2]
if player_position[0][1] == 4:
del moves[3]
if player_position[0][0] == 4:
del moves[1]
if player_position[0][0] == 0:
del moves[0]
return moves
def start():
monster = random.sample(CELLS, 1)
door = random.sample(CELLS, 1)
player = random.sample(CELLS, 1)
return monster, door, player
def start_message():
print('welcome to the dungeon!')
print('This map is 5x5 grid')
print('enter QUIT to quit')
start_message()
monster = start()[0]
door = start()[1]
player = start()[2]
winstreak = 0
#start of loop ===============================
while True:
if player == None:
monster = start()[0]
door = start()[1]
player = start()[2]
print('You are currently in row {} and colum {}'.format(player[0][1]+1, player[0][0]+1))
print('you can move{}'.format(unpacking_list(get_moves(player))))
print('. . . . .\n. . . . .\n. . . . . \n. . . . . \n. . . . .\n\n\n\n\n\n\n\n\n\n\n\n')
move = input('> ')
move = move.upper()
if move == 'QUIT':
break
if any(move in s for s in get_moves(player)):
player = move_player(player, move)
player = [tuple(player)]
else:
print('\n\nError. It may be that you went out of the grid, or you wrote wrong\n')
if player == door:
winstreak += 1
print('GJ you won! How big winstreak can you get\nWinstreak: {}'.format(winstreak))
player = None
start_message
continue
if player == monster:
winstreak += 0
print('Nooo, you met the monster. You lost, your winstreak stopped, but keep trying!\nWinsteak: 0')
player = None
start_message
continue
it's long, so it's maybe easier if you copy it over to your text editor.
6 Answers
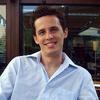
Pedro Cabral
33,586 PointsFew observations from my side:
1. Your method unpack_list(list) is redundant as you can do the same with * for example write the following and see the results:
print(*["a","b","c"])
- Avoid using reserved keywords as function arguments such as list.
2. This line print('. . . . .\n. . . . .\n. . . . . \n. . . . . \n. . . . .\n\n\n\n\n\n\n\n\n\n\n\n') would me more readable if you would use a string literal/triple-quotes:
print(
""". . . . .
. . . . .
. . . . .
. . . . .
. . . . .""")
3. Python is a very human-readable language, in my opinion comments like this:
#start of loop ===============================
just clutter the code, anyone reading
while True
will understand that that's the start of a loop.
4. Your winning streak logic is faulty, if I win 2 times straight, fail 1 and then win 1, my winning streak should be 2 but it shows as 3. So at the moment is showing how many times I have won instead of the biggest streak.
5. This does nothing.
winstreak += 0
It's the same as winstreak = winstreak + 0, so you are setting the variable value to the value that it already holds. After that you follow it with the following line:
print('Nooo, you met the monster. You lost, your winstreak stopped, but keep trying!\nWinsteak: 0')
Which is also not true, if the winstreak is 2, the print will "lie" saying it's zero when it's not. For accurate information use the value that the variable holds instead of trying to guess as you might run into bugs such as this.
6. Finally you have a few typos.
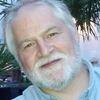
Jeff Muday
Treehouse Moderator 28,722 PointsPedro Cabral has some excellent suggestions.
I like your code-- that you took the time to show it is a great step! Keep up the good work and maybe starting posting your code to GitHub.
Pedro and I would probably agree that on comments: never sacrifice clarity for brevity. I thought it was appropriate to comment where your main program loop was-- get in the habit of planning, commenting, and refactoring. At some point you'll be presenting a GitHub or Bitbucket portfolio to a potential employer; thoughtful descriptions/comments in your README and in-code notes may become interview topics.
Here's a pretty funny/true article (while it's about PHP, it might as well be about any other language):
https://code.tutsplus.com/tutorials/why-youre-a-bad-php-programmer--net-18384
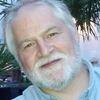
Jeff Muday
Treehouse Moderator 28,722 PointsGithub and Bitbucket are repositories and can be used as a code versioning system. And now, Github in particular, can be used as a website for code (there is a trend to use Github site as part of an aspiring developer's portfolio and sometimes as an entire resume website). Treehouse has instruction on Github-- I recommend you take it!
One cool Github feature is you can "follow" a developer. That is, you can get notifications of some of their high-level Github actions/activities.
Here is Kenneth Love's site: https://github.com/kennethlove
In essence, a developer will put their code up there to show/share with others and sometimes work on a team project (I contribute to several projects). More than that, Github can be used during the development process to maintain consistent versioning among team members. Github also will allow an individual to "fork" code of others. By "fork", we mean one can copy an entire project and begin a new branch of the code to improve or change it while leaving the original code version unchanged.
Bitbucket is similar to Github, it is not as full-featured, but the advantage of Bitbucket is you can keep your code private (for free). With Github you have to pay to keep your code secret. I hide quite a bit of my code that I write because it was written for my employers or side projects and wasn't intended to be in the public domain.

Pitrov Secondary
5,121 PointsThanks for the comment Jeff Muday, I maybe watch through the Github course.

Pitrov Secondary
5,121 PointsThank you, Pedro Cabral and Jeff Muday. You taught me much, I didn't know that I could unpack lists with a *, and I will start using comments better.
I've heard about GitHub, but I've never tried it. How does Github work? Do people just help me with my code?
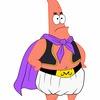
<noob />
17,062 PointsHi, can u explain what this line of code do?
f player_position[0][1] == 0:
del moves[2]
if player_position[0][1] == 4:
del moves[3]
if player_position[0][0] == 4:
del moves[1]
if player_position[0][0] == 0:
del moves[0]
return moves
how exactly ur accessing the cells? how u determine which direction is it?
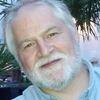
Jeff Muday
Treehouse Moderator 28,722 Pointsdskellth theSmartGuy is the programmer of this version, so with luck he will respond to you! Being able to explain your code to others really is a good step toward mastery of Python.

Pitrov Secondary
5,121 Pointsnoob developer. First, of, I am sorry that my code is white, I don't know how to make it with colors. My function get_moves(player_position) checks all the possible directions my player can go. It takes the position of my player as a parameter, which is 'player'. 'Player' is a list of a tuple like [(1, 0)] for example. In the first line, I checked if player_position[0][1] is equal to 0. player_position[0][1] is just the first list and the second item in the tuple. if it is 0 like it is in the example, then I can't go up, so I delete the 3rd item in the list 'moves'. which has an index of 2. then I check if playerposition[0][1] is 4. if it was then I couldn't go down. I would have had to delete the 4th item in the list 'moves', which has the index of 3. Then I do the same to the first item in the tuple. Lastly, I return moves, which now contains moves that the player can go, so that he doesn't fall out of the map.
I hope you understand what I am talking about.
def get_moves(player_position):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN',]
if player_position[0][1] == 0:
del moves[2]
if player_position[0][1] == 4:
del moves[3]
if player_position[0][0] == 4:
del moves[1]
if player_position[0][0] == 0:
del moves[0]
return moves
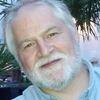
Jeff Muday
Treehouse Moderator 28,722 PointsTo make your code syntax colored, use three backticks the word python and then end code segment with three backticks. Keep up the good work!
def get_moves(player_position):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN',]
if player_position[0][1] == 0:
del moves[2]
if player_position[0][1] == 4:
del moves[3]
if player_position[0][0] == 4:
del moves[1]
if player_position[0][0] == 0:
del moves[0]
return moves