Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial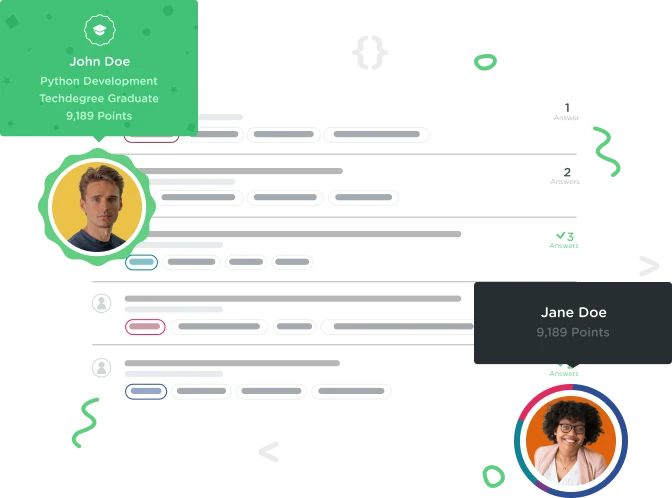

Ulyssa Sneed
8,894 PointsI might not be smart enough for C# smh
using System;
class Program
{
static string quote(string phrase)
return
// YOUR CODE HERE: Define a Quote method!
static void Main(string[] args)
{
// Quote by Maya Angelou.
Console.WriteLine(Quote( \"When you learn, teach. When you get, give.\""));
// Quote by Benjamin Franklin.
Console.WriteLine(Quote(/"No gains without pains./""));
}
}
1 Answer
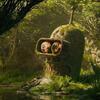
Samuel Tredgett
11,372 PointsHi Alyssa, there are a couple of problems you've got here but hopefully we can correct them and let you know where the misunderstanding is.
Firstly, in your method definition for the Quote method there are a few mistakes. You should try to always capitalise the first letter of a method. The second issue with creating the class is that you've forgotten the '{}' curly braces that contain the code the method executes. If you wanted to just build the method without it doing anything you'd write it as so:
static string Quote(string phrase) {
//Your code here
}
You've also tried to implement the logic of the string escape characters into the Main method, which isn't what we want to do here. Remember, the point of writing a method is so that you reduce repeated code and simplify your own life when working on projects. In your example you've written:
// Quote by Maya Angelou.
Console.WriteLine(Quote( \"When you learn, teach. When you get, give.\""));
// Quote by Benjamin Franklin.
Console.WriteLine(Quote(/"No gains without pains./""));
The problem with this is a couple of small but fixable things. We don't need to be adding in the escape character '\' as we're going to implement that functionality into the Quote method. The only thing needed here in Main is the Console.WriteLine method which passes in as an argument a Quote method call on the string we wish to put inside quotation marks. What we're doing here is manipulating the fact that the Quote method will return the string we give it as a String with speech marks at each side. So as far as Main is concerned, it only needs to write out whatever Quote is going to print. Thus we can write it like this:
// Quote by Maya Angelou.
Console.WriteLine(Quote("When you learn, teach. When you get, give."));
// Notice how the Quote method call is nested inside the Console.WriteLine method call
// Quote by Benjamin Franklin.
Console.WriteLine(Quote("No gains without pains."));
Lastly, now that we're calling the method properly again we just need to implement the escape sequence functionality into that method. Here we can make use of String interpolation in order to achieve this more neatly. See the example code snippet below for a working version:
static string Quote(string phrase) {
//Your code here
return $"\"{phrase}\"";
}
To explain what's going on here, recall that the '\' symbol inside the context of speech marks provides special interactions with the next character that appears. In this example we want to add extra speech marks, therefore we write '\"' inside of them.
On top of that we've added interpolation. Interpolation makes use of the '$' symbol before the start of a string and then allows you to pass a variable into that string in order for it to be printed as part of the string. Here I've used '{phrase}' so that when this method returns the string, the phrase string we gave it as an argument is inserted between the special '\"' escapes we made to put quotation marks around it. This allows us to complete our fully fledged Quote method and solve the problem at hand.
After running all of this we then get the return:
"When you learn, teach. When you get, give."
"No gains without pains."
I might not be smart enough for C# smh Programming is difficult at first but it does get easier! Stick at it and you'll be here answering questions before you know it :) Best of luck.
Scott Kim
518 PointsScott Kim
518 PointsThis is the greatest - thank you!