Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial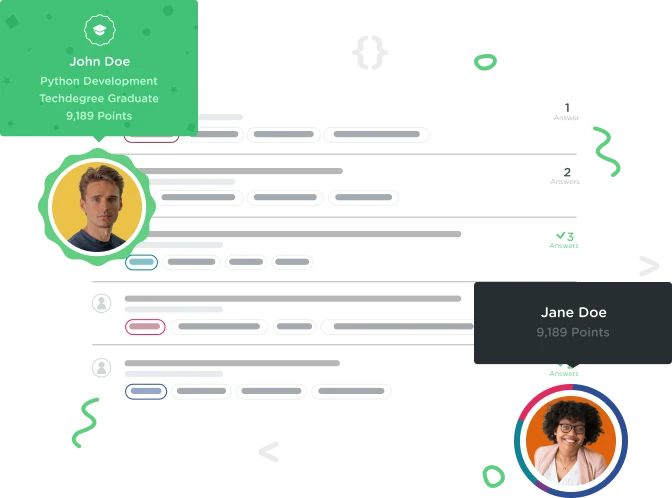

Daniel Lee
Courses Plus Student 272 PointsI need clarifications on these terms exception and directives @Try, @finally, @throw.
What is the general reason an exception occur? When an exception appears, does the program terminate immediately or keep running?
I'm guessing @try is the program message I'm testing. Is that right?
After @catch either log an error message, clean up, etc, what does the @finally block do? The book I'm reading says that @finally determines whether or not a statement in an @try throws an exception. But isn't that an unnecessary step because with or without it, we could tell whether there was an exception based on the program terminating abruptly?
What's the throw directive? The books says it enables you to throw your own exception. But I find that really confusing. Does that mean I could somehow create an exception and test it out?
I would really appreciate it if you could answer at least one of the questions for me. Thank you.
1 Answer
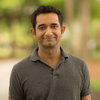
Amit Bijlani
Treehouse Guest TeacherFirstly, the try/catch/finally approach is not a preferred approach in Objective-C - you simply do not need it as libraries seldom throw exceptions. The widely used convention is that of a delegate which has an error method callback, or perhaps notifications for more general failures.
That said. Here's a general explanation for try/catch/finally. Let's assume we have a custom subclass of NSArray
and have a method called createSubclassOfArray
to initialize and allocate that array.
- (id) createSubclassOfArray {
@try {
// try to allocate and initialize custom array
// return subclass of NSArray
}
@catch (NSException *theException) {
// Catches the exception and throws it to the caller of this method
@throw; // implicitly throws theException
}
}
// somewhere else in your code
id array;
@try {
// Try to create the custom array
array = [self createSubclassOfArray];
}
@catch (NSException *theException) {
// couldn't create custom array so just create NSMutableArray
array = [NSMutableArray array];
}
@finally {
// No matter what happens add an item to the array
// This block executes whether or not there was an exception
[array addObject:@"First Item"];
}