Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial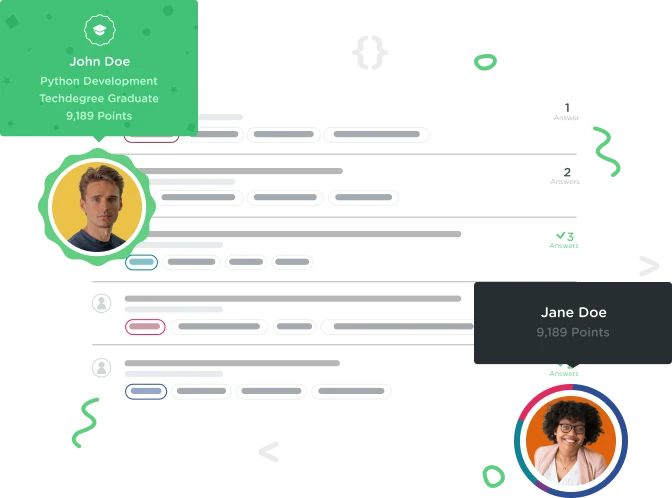

Gilang Ilhami
12,045 PointsI need explanation
# make a ist that wil hold on to our items
shopping_list = []
# print out the instructions on how to use the app
print ("What should we do today?")
print ("Type DONE when you want to quit.")
while True:
# ask for new items
new_items = input("> ")
# be able to quit the app
if new_items == 'DONE':
break
# add new items to our list
shopping_list.append(new_items)
# print out the list
print ("Here is your To Do list!")
for items in shopping_list:
print(items)
#This script above ends up with the same results as what were in the video
#But i did some little mistake, and the result intrigue me. With the script below i will get
#(Here is your list:
#Apple
#Car
#DONE)
#Can someone explain to me why? This is very interesting
# make a ist that wil hold on to our items
shopping_list = []
# print out the instructions on how to use the app
print ("What should we do today?")
print ("Type DONE when you want to quit.")
while True:
# ask for new items
new_items = input("> ")
# add new items to our list
shopping_list.append(new_items)
# be able to quit the app
if new_items == 'DONE':
break
# print out the list
print ("Here is your To Do list!")
for items in shopping_list:
print(items)

fahad lashari
7,693 PointsYou need to basically swap around these two and add an else statement to your if statement:
#add new items to our list
shopping_list.append(new_items)
#be able to quit the app
if new_items == 'DONE':
break
check to see if new_items == 'DONE' before appending it to the shopping_list:
#be able to quit the app
if new_items == 'DONE':
break
else:
#add new items to our list
shopping_list.append(new_items)
This way 'DONE' does not get added to the list and the loop breaks before adding DONE to the list.
Do let me know if this helped
Kind regards
1 Answer

Benjamin Lange
16,178 PointsThe difference that I see between these two blocks of code is the order of the ask for new items block and the be able to quit the app block.
In the first version, you check the new_item BEFORE you add it to the list to make sure that it isn't "DONE". If the new_item is equal to "DONE", then you're immediately breaking out of the while loop and skipping over any code that is left in the while loop. "DONE" will not get a chance to be added to the shopping_list.
I the second version, you add new_item to shopping_list no matter what. You're not checking to see if new_item is equal to "DONE" first. You add new_item to the list and then check to see if new_item is equal to "DONE". If it is, you break out of the while loop, but "DONE" was already added to the shopping list.
As you can see from your example, a slight change in the order of your code can make a huge difference.
Nate Sturgeon
2,255 PointsNate Sturgeon
2,255 PointsPlease format your code so it is easier to read. Also, we can't see your indentation without the proper formatting, which is often the cause of errors. To format your code, enclose all of it in 3 backticks (
) followed by the word python above and another 3 backticks (
) below:It should look like this:
and will display like this to the rest of us: