Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial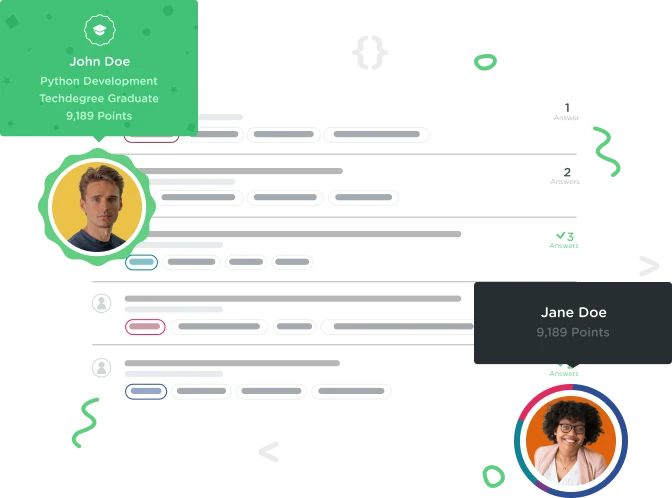
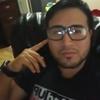
Frank Campos
4,175 PointsI need help !!!!
I dont undertans how answer[0] can be True?
import datetime
import random
from timed_quiz_app import Add, Multiplication
class Quiz:
question = []
answers = []
def __init__(self):
questions_type = (Add, Multiplication)
for _ in range(10):
question = random.choice(questions_type)
num1 = random.randint(1, 10)
num2 = random.randint(1, 10)
self.question.append(question(num1, num2))
# random questions with numbers from 1 to 1o self.question = quesiton
# add this question to self.Questions self.Questions.append(self.question)
def take_quiz(self):
self.start_time = datetime.datetime.now() # log the start time self.start_time = time.time()
for question in self.question: # ASK THE QUESTION
self.answers.append(self.ask(question)) # log if we have the questions right (True or False, Seconds)
self.end_time = datetime.datetime.now() # log the end time
return self.summarie() # show a summary
@staticmethod
def ask(question):
question_start = datetime.datetime.now() # log the start time
answer = input(question.text + '= ') # capture the answers
if answer == str(question.answer):
correct = True # check the answer
print('good')
else:
correct = False
print("bad")
question_end = datetime.datetime.now() # log the end time
return correct, question_end - question_start # if the answer is right sent back True
# otherwise send back False
# send back the elapsed time
def total_correct(self):
total = 0
for answer in self.answers:
if answer[0]:
total += 1
return total
def summarie(self):
print('You got {} out of {} right'.format(self.total_correct(), len(
self.question))) # print how many had right and de total of the questions
print("It took you {} second total".format((self.end_time - self.start_time).seconds))
Quiz().take_quiz()
5 Answers

ds1
7,627 PointsGotcha. Had the same question on a script I wrote before this one. Ok, this if statement is triggered if its expression is True, right? But I guess all the if statement really needs is the expression's value... so you could write "if True:" if you wanted an if statement that always was True. Try it in your IDLE... it works. Well, if answer[0] is "True" or "False", then you can just write "if answer[0]:". But let's take it a step further.... if I have a variable named "arg" and it was set to None... or it was 0 or it was an empty string, then if you typed "bool(arg)" it would return False. So you could also write "if arg:" if you wanted your code to do something only when "arg" had a truthy (as Kenneth likes to say) value.

ds1
7,627 PointsHi, Frank Here's some of my code for this video:
import datetime
import random
from questions import Add, Multiply
class Quiz:
questions = []
answers = []
def __init__(self):
question_types = (Add, Multiply)
# generate 10 random questions with numbers from 1 to 10
for _ in range(10):
num1 = random.randint(1, 10)
num2 = random.randint(1, 10)
question = random.choice(question_types)(num1, num2)
# add these questions into self.questions
self.questions.append(question)
def take_quiz(self):
# log the start time
self.start_time = datetime.datetime.now()
# ask all of the questions
for question in self.questions:
# log if they got the questions right
self.answers.append(self.ask(question))
else:
# log the end time
self.end_time = datetime.datetime.now()
# show a summary
return self.summary()
def ask(self, question):
correct = False
# log the start time
question_start = datetime.datetime.now()
# capture the answer
answer = input(question.text + ' = ')
# check the answer
if answer == str(question.answer):
correct = True
# log the end time
question_end = datetime.datetime.now()
# if the answer's right, send back True
# otherwise, send back False
# send back the elapsed time too
return correct, question_end - question_start # Notice that a tuple is being returned
def total_correct(self):
# return the total number of correct answers
total = 0
for answer in self.answers:
if answer[0]:
total += 1
return total
def summary(self):
# print how many were answered correctly and the total number of questions
print('You got {} out of {} right.'.format(
self.total_correct(), len(self.questions)
))
# print the total time for the quiz
print('It took you {} seconds total.'.format(
(self.end_time-self.start_time).seconds
))
# Take a quiz
Quiz().take_quiz()

ds1
7,627 PointsYou see, the ask method returns a tuple, with the first item being "True" if the answer was correct. Now check out the take_quiz method. This method runs the game, using other methods and attributes as it needs. Note this section of code:
# ask all of the questions
for question in self.questions:
# log if they got the questions right
self.answers.append(self.ask(question))
the answers attribute holds the return value of the ask method... so that means that "answers" is a list of tuples... you'll want to remember that.
So when the total_correct method below is run...
def total_correct(self):
# return the total number of correct answers
total = 0
for answer in self.answers: # check out this line
if answer[0]:
total += 1
return total
for "answer" (i.e. for each item [which is a tuple that starts with either True or False based on if the user got the right answer] in the "answers" attribute) if "answer[0]" (i.e. if that first item in the tuple is True) then increment the total. The total_correct method eventually returns the total variable of how many the user got right. Hope that helps! D.
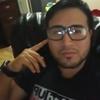
Frank Campos
4,175 PointsThank you a lot, ds1 !!! so what happened is the answer of the tuple is False?? I do understand that the answer[0] is asking for the first value of tuple. However, the part I don't understand is that we are not telling explicitly that answer[0] == True do this? don't we need it in this case to do it?
Thank you for quick answer by the way!!!
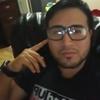
Frank Campos
4,175 PointsThanks ds1 , this part is a little confusing, but it is the way how python works. To wrapping this doubt up when I find something like 'if arg[0]:' is saying if this condition is only True do this. Well now, I have to fill those gaps in my knowledge about how the booleans work.
Thank you for your time, I hope to see around here more often.

ds1
7,627 PointsThanks, Frank! Fyi, I think you could write "if arg[0]== True:" (I'm not at my computer to double-check this) but you don't have to. Plus, now you know what's going on when you see Kenneth or others writing it this way ; )