Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial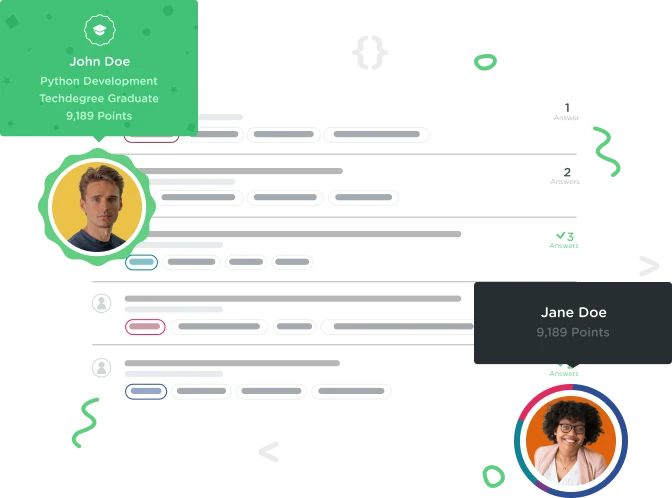

Unsubscribed User
Courses Plus Student 2,233 PointsI need help
I did not understand what he wanted
// Declare protocol here
protocol ColorSwitchable {
func switchColor ()
}
enum LightState {
case on, off
}
enum Color {
case rgb(Double, Double, Double, Double)
case hsb(Double, Double, Double, Double)
}
class WifiLamp {
let state: LightState
var color: Color
init() {
self.state = .on
self.color = .rgb(0,0,0,0)
}
}
1 Answer

Jasper De Ricci, Carafa
4,803 PointsHi Mohammad,
The code challenge is asking you to create a protocol named ColorSwitchable and write a function inside the protocol called switchColor. The function should have an underscore ( _ ), used to denote a blank in the place of the external name, and color as the local name of the parameter. The underscore is its own symbol and MUST be separated from all other symbols. The code challenge also requires that you specify an argument, Color, which is written after the local name of the function parameter and separated by a colon. The second task of the code challenge requires you to copy the switchColor function into the class WifiLamp so that WifiLamp adopts the ColorSwitchable protocol you wrote earlier. Inside the function, simply set the color to itself using the self keyword. For WifiLamp to conform to ColorSwitchable, write ColorSwitchable after the the class WifiLamp using the same technique as in class inheritance.
// Declare Protocol Here
// Task 1
protocol ColorSwitchable {
func switchColor ( _ color: Color )
}
enum LightState {
case on, off
}
enum Color {
case rgb(Double, Double, Double, Double)
case hsb(Double, Double, Double, Double)
}
class WifiLamp: ColorSwitchable {
let state: LightState
var color: Color
init() {
self.state = .on
self.color = .rgb(0, 0, 0, 0)
}
func switchColor ( _ color: Color ) {
self.color = color
}
}