Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial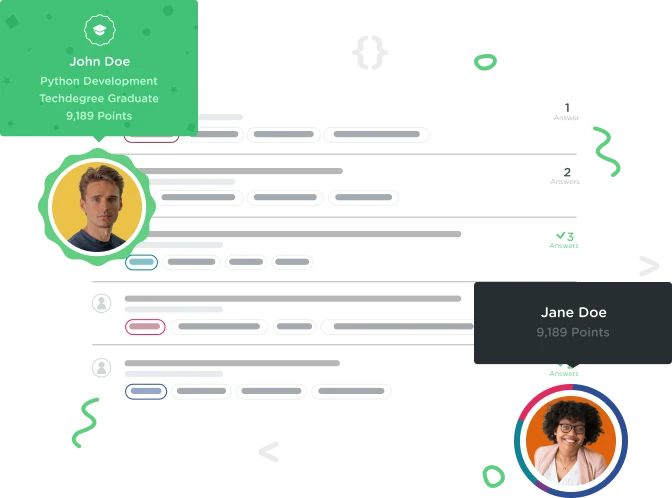

Natalia Henderson
5,190 PointsI Need Help!
class Base: def init(self, name, color, texture, **kwargs): self.name = name self.color = color self.texture = texture
from base import Base class Clay(Base): def init(self, malleable = True): super().init(name, color, texture, **kwargs) self.malleable = malleable
from base import Base from special_attributes import Clay gravel = Clay(malleable = True, name = "Clay", color = "grey", texture = "smooth")
SyntanxError - pointing at **kwargs in init in Base class
TypeError: init() got multiple values for argument ‘malleable’
TypeError: init() got an unexpected keyword argument ‘name’
I ran it multiple times, so some of the errors may not be relevant. Thank You!
2 Answers
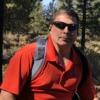
Mark Kastelic
8,147 PointsHi Natalia,
After you copy and paste your code into the text box, type: ```python on the line just above the first line of code and then close with ``` on the line following your last line of code. Note: These three tick marks ``` are not single quotes, but are found on the same key as the tilde ~ (see the Markdown Cheatsheet ). I have entered your modules below along with what I believe to be the corrections needed to make your code work:
base.py
class Base:
def __init__(self, name, color, texture, **kwargs):
self.name = name
self.color = color
self.texture = texture
special_attributes.py
from base import Base
class Clay(Base):
def __init__(self, name, color, texture, malleable = True, **kwargs):
super().__init__(name, color, texture, **kwargs)
self.malleable = malleable
main.py
from base import Base
from special_attributes import Clay
gravel = Clay(malleable = True, name = "Clay", color = "grey", texture = "smooth")
In summary, you must use "__init__" (dunder init) instead of just "init" everywhere in your code. This is the special named method that Python knows to call automatically on the creation of an instance object. Yes, super( ) will override the __init__ in class Clay and use the parent Base __init__, but parameters still have to be defined for the Clay object in order to be passed back to Base. This ran without error and I could access all the attributes of the gravel object correctly. Hope this helps.
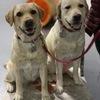
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Mark,
Natalia was using dunder init, but the markdown conversion treats text outside of a code block wrapped with dunders as a bold specifier, hence her inits are bold in the question.
Cheers
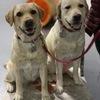
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Natalia,
Because of the mangling applied during the HTML conversion and sanitization, I can't be sure that this covers all the possible issues with your code but here are a couple of items that jumped out at me:
Your Clay.__init__
does not have a **kwargs
argument but you are referring to kwargs
in the body of your method.
You are calling the Clay.__init__
method when you create gravel
but you are passing keyword arguments (name
, color
, and texture
) without either having those as defined parameters in your initialiser or having a **kwargs
parameter to accept arbitrary keyword arguments.
Hope that helps. Cheers,
Alex
Alex Koumparos
Python Development Techdegree Student 36,887 PointsAlex Koumparos
Python Development Techdegree Student 36,887 PointsHi Natalia,
Please ensure that you always apply proper markdown formatting when putting code in a question. There is a link to the Markdown Cheatsheet below each text entry box if you forget the proper syntax).
This is important in all languages but especially important for Python where the conversion to HTML will completely mangle your code and make it essentially impossible to debug thoroughly.
Cheers,
Alex