Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial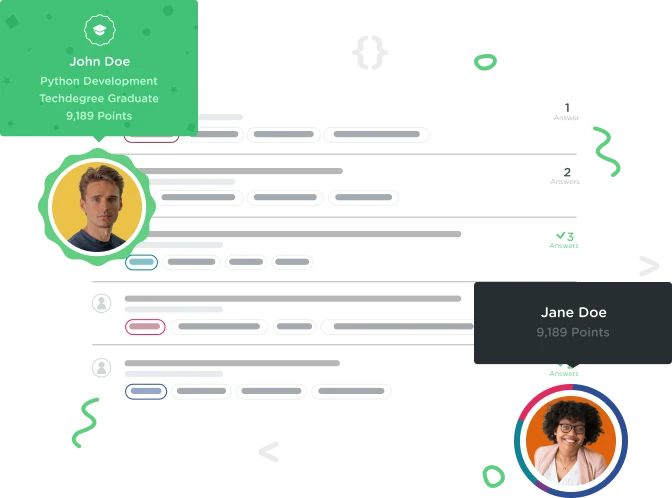

Gem Knight
4,779 PointsI need help
I am trying to replicate the TreeHouse frog fitness program in my own way in workspaces. However, I am not sure where and how to do a while loop in my code, so that the code block will repeat until the user types in 'n'. Can anybody please help me.
This is my code so far:
using System;
class Program
{
static void Main()
{
Console.WriteLine("Are you ready to start working out: (y/n)");
string readyTo = Console.ReadLine();
if (readyTo == "y")
{
Console.WriteLine("Good job! Get ready to workout...");
}
else
{
Console.WriteLine("Too bad you are working out anyway! So get your towel ready.");
}
Console.WriteLine("Start working out....NOW! \nRecord your minutes when ready.");
Console.WriteLine("How many minutes have you worked out...");
string minWorkOut = Console.ReadLine();
int startTime = 0;
int WorkOut = int.Parse(minWorkOut);
int totalTime = WorkOut + startTime;
Console.WriteLine(totalTime + " is how many minutes you have worked out so far.");
Console.WriteLine("Do you want to add more minutes: (y/n)");
string answer = Console.ReadLine();
if (answer == "y")
{
Console.WriteLine("Keep up the good work!");
Console.WriteLine("Record the amount of minutes when you're ready.");
string amountOM = Console.ReadLine();
int amountOMI = int.Parse(amountOM);
totalTime = totalTime + amountOMI;
Console.WriteLine(totalTime + " is the amount of time you have done now.");
}
else
{
Console.WriteLine("Alright. bye, thanks for using this program.");
}
}
}
1 Answer
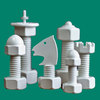
Steven Parker
230,274 PointsYou forgot to provide a link to the course, but didn't the course show you how to construct a loop? You might want to go back to the course and see if there's a section about loops you can review.
But about your question, besides needing a loop, I noticed a few other things:
- you've replicated some code you can re-use in the loop
- you don't need that startTime variable.
- you need to add WorkOut to TotalTime
- you should preset TotalTime to 0 before the loop
- you need a using System directive for the Console calls you have
You probably want something like this:
using System;
class Program
{
static void Main()
{
Console.WriteLine("Are you ready to start working out: (y/n)");
string readyTo = Console.ReadLine();
if (readyTo == "y")
{
Console.WriteLine("Good job! Get ready to workout...");
}
else
{
Console.WriteLine("Too bad you are working out anyway! So get your towel ready.");
}
int totalTime = 0;
string answer;
do {
Console.WriteLine("Start working out....NOW! \nRecord your minutes when ready.");
Console.WriteLine("How many minutes have you worked out...");
string minWorkOut = Console.ReadLine();
int WorkOut = int.Parse(minWorkOut);
totalTime += WorkOut;
Console.WriteLine(totalTime + " is how many minutes you have worked out so far.");
Console.WriteLine("Do you want to add more minutes: (y/n)");
answer = Console.ReadLine();
} while (answer != "n");
Console.WriteLine("Alright. bye, thanks for using this program.");
}
}