Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial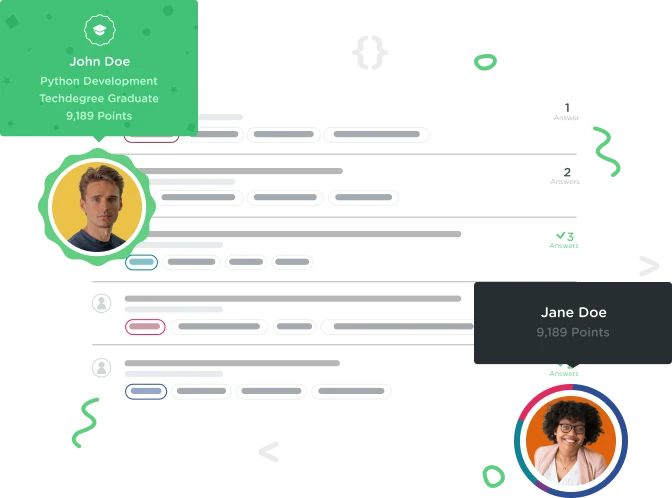

Joseph Dicks
1,860 PointsI need help getting rid of the title bar using Android Studio
I can not get rid of the title bar at the type, I was wondering if someone could help me on my code.
maybe @Ben Jakuben can help me?
I have modified my activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/black"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
tools:context=".MainActivity">
<ImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/imageView"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:src="@drawable/ball01"
android:scaleType="fitCenter"
android:layout_gravity="center" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/textView"
android:layout_centerInParent="true"
android:layout_marginLeft="65dp"
android:layout_marginTop="135dp"
android:textSize="32sp" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Enlighten me"
android:id="@+id/button"
android:layout_below="@+id/textView"
android:layout_centerHorizontal="true" />
</RelativeLayout>
I have modified my styles.xml
<resources>
<!--
Base application theme, dependent on API level. This theme is replaced
by AppBaseTheme from res/values-vXX/styles.xml on newer devices.
-->
<style name="AppBaseTheme" parent="Theme.AppCompat.Light">
<!--
Theme customizations available in newer API levels can go in
res/values-vXX/styles.xml, while customizations related to
backward-compatibility can go here.
-->
</style>
<!-- Application theme. -->
<style name="AppTheme" parent="AppBaseTheme">
<!--
All customizations that are NOT specific to a particular API-level can go here.
-->
<item name="android:windowNoTitle">true</item>
<!-- Hides the Action Bar -->
<item name="android:windowFullscreen">true</item>
<!-- Hides the status bar -->
</style>
</resources>
I have modified my main activity.java also.
package com.example.crystalball;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends Activity {
private CrystalBall mCrystalBall = new CrystalBall();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Declare our View Variables and assign them the Views from the layout file
final TextView answerLabel = (TextView) findViewById(R.id.textView);
Button getAnswerButton = (Button) findViewById(R.id.button);
getAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String answer = mCrystalBall.getAnAnswer();
// Update the label with our dynamic answer
answerLabel.setText(answer);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
3 Answers
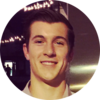
Connor Myers
21,089 PointsIf you are trying to hide the action bar at the top of the app you would use this code:
ActionBar actionBar = getActionBar();
actionBar.hide();
You are going to need to optimize your imports and if you are in a fragment activity then the code is going to be a little different! Hopefully this helps!

Joseph Dicks
1,860 PointsConnor,
Thanks for the response. I'm assuming that goes in the main activity.java file?
Joe
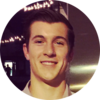
Connor Myers
21,089 PointsCorrect! You can hide the action bar in any activity that you have but you have to do it in the respective activity in which you are wanting to hide the action bar.

Joseph Dicks
1,860 PointsConnor,
Thanks for the help!
Joe