Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial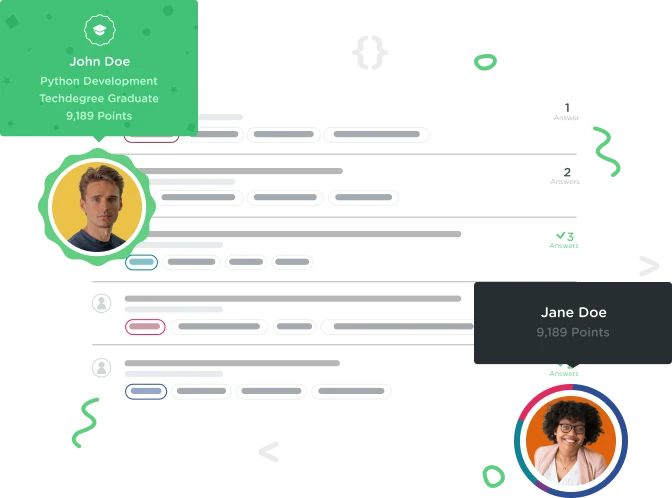

Karl Bjorkman
1,808 PointsI need help here too. What is wrong with my init method in my subclass?
Any suggestions will be much appreciated. Thanks!
class Vehicle {
let wheels: Int
let doors: Int
// Designated initializer
init(wheels:Int, doors:Int){
self.wheels = wheels
self.doors = doors
}
}
class Car: Vehicle {
// A car must default to 4 wheels and 4 doors
init(wheels: Int = 4, doors: Int = 4){
super.init()
}
}
1 Answer
William Li
Courses Plus Student 26,868 PointsThere're 3 lines of comments on the code template provided by the Code Challenge, and below each comment, there's code you are supposed to fill in.
1st comment - // Designated initializer
, no problem here, you got it right.
2nd comment - // A car must default to 4 wheels and 4 doors
, you got it mostly correct, only that you're missing the override keyword, this keyword is needed to override the same method from its parenting class.
3rd comment - // call super.init
. You are correct to call in the super.init()
to do the job, however, problem is that this method takes 2 arguments, there's none in your code. It's true that the overrided init method in the Car class has the default values for both wheels and doors; but only when creating a new car object are you allowed to not passing these 2 arguments along to the constructor; when writing the class definition, however, you don't have that luxury.
Now, to put everything together. The corrected version of your code should look sth like this.
class Vehicle {
let wheels: Int
let doors: Int
// Designated initializer
init(wheels:Int, doors:Int){
self.wheels = wheels
self.doors = doors
}
}
class Car: Vehicle {
// A car must default to 4 wheels and 4 doors
override init(wheels: Int=4, doors: Int=4){
// call super.init
super.init(wheels: wheels, doors: doors)
}
}