Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial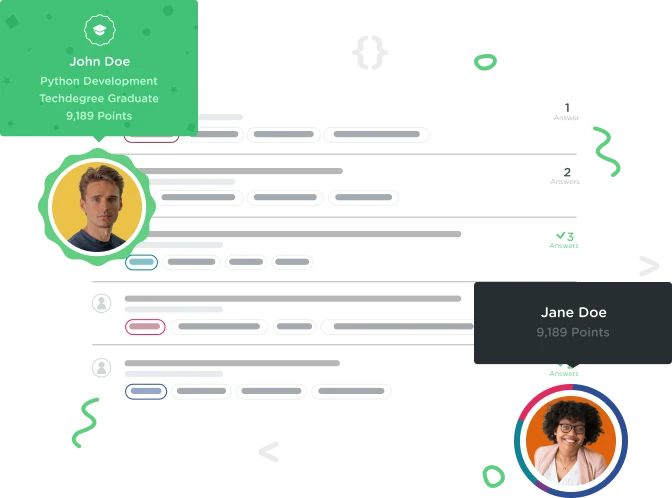

walter fernandez
4,992 PointsI need help, I don't know where the error is.
Alright, this one might be a bit challenging but you've been doing great so far, so I'm sure you can manage it. I need you to make a function named word_count. It should accept a single argument which will be a string. The function needs to return a dictionary. The keys in the dictionary will be each of the words in the string, lowercased. The values will be how many times that particular word appears in the string.
"The code has to give me a dictionary and the number of times that the value repeat " example word_count("I go to the school when I want") {'I':2, 'go': 1, 'to':1, 'the':1, 'school':1, 'when' : 1,' want':1 }
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(t):
s = t.lowercase()
4 Answers
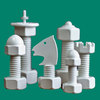
Steven Parker
229,732 PointsOther than fixing the function name ("lower") you'll need a good bit more code after that one line to meet the objectives. Here's some hints:
- now that you have the string in lower case, you'll probably want to break it up into individual words
- you'll need to create a dictionary to build the result in
- a loop would be handy to step through the individual words
- for each word you will either add it to the dictionary, or increase the count if it's there
- after the loop, you'll "return" the newly created dictionary
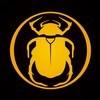
rydavim
18,813 PointsOkay, so just in terms of the current error...
def word_count(t):
s = t.lowercase() # The syntax here should be .lower()
However, fixing that will still give the general "Bummer: Try again!", so let me know if you're still stuck after that and we can go through some pseudo-code or a possible solution. I haven't done this particular course yet, so keep in mind that there are multiple ways to solve this challenge.
This is just a general tip, but I would also recommend using more descriptive variable names. Particularly in the beginning, I think it helps keep track of everything. But that is personal preference, and certainly not a requirement.
Happy coding!

walter fernandez
4,992 Pointssorry i meant where is the error in this code ? the problem is the same. this code
def word_count(t):
r = t.lower()
a = r.split(",")
for value in a.values():
return value
for i in value():
if value[] == value[]:
return a
for item in r.items():
print(item)
Mod Edit: Wrapped code in back-ticks for formatting.
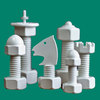
Steven Parker
229,732 PointsDid you resolve this already? But in case not, here's a few more specific hints (the others still apply):
- you'll want to use "
split()
" with no arguments so it will handle all white space - you will probably only need one loop
- you won't want to "return" until the loop finishes
- you do not need to "print" anything

walter fernandez
4,992 Pointssorry, for the quality of my last post, I posted the question again, I hope you guys can help me.
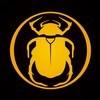
rydavim
18,813 PointsPython isn't my strongest language, so hopefully Steven Parker can take a shot at it. I'll check back in a bit later and take a look if needed.
I've formatted the code in your second post so it's easier to read. To do this yourself, simply wrap your code in three back-ticks and add a language for fancy syntax highlighting.
```python
Your code here!
```
# Your code here!