Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial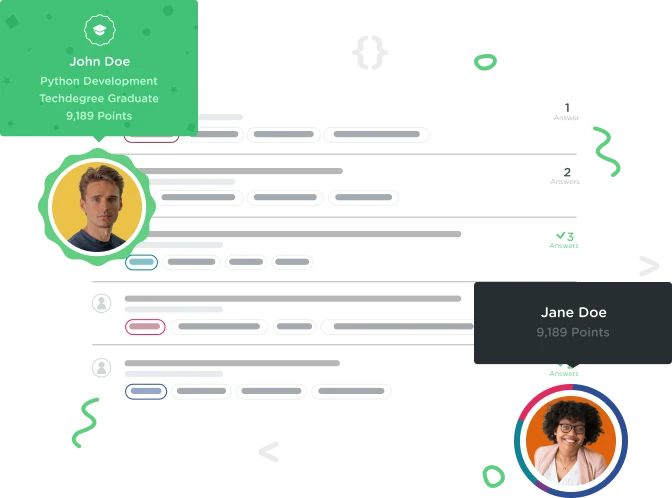

a a
1,101 PointsI need help in code challenge
Python 3.6.3 |Anaconda, Inc.| (default, Oct 15 2017, 03:27:45) [MSC v.1900 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> def disemvowel(word):
... word_1 = list(word)
... for words in word_1:
... if "a" in words:
... words.remove("a")
... if "A" in words:
... words.remove("A")
... if "i" in words:
... words.remove("i")
... if "I" in words:
... words.remove("I")
... if "u" in words:
... words.remove("u")
... if "U" in words:
... words.remove("U")
... if "e" in words:
... words.remove("e")
... if "E" in words:
... words.remove("E")
... if "o" in words:
... words.remove("o")
... if "O" in words:
... words.remove("O")
... word_2 = ",".join(words)
... print(word_2)
...
>>> disemvowel("HUBxcbeYq")
H
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<stdin>", line 15, in disemvowel
AttributeError: 'str' object has no attribute 'remove'
>>>
def disemvowel(word):
word_1 = list(word)
for words in word_1:
if "a" in words:
words.remove("a")
if "A" in words:
words.remove("A")
if "i" in words:
words.remove("i")
if "I" in words:
words.remove("I")
if "u" in words:
words.remove("u")
if "U" in words:
words.remove("U")
if "e" in words:
words.remove("e")
if "E" in words:
words.remove("E")
if "o" in words:
words.remove("o")
if "O" in words:
words.remove("O")
word_2 = ",".join(words)
print(word_2)
1 Answer
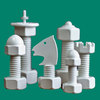
Steven Parker
231,269 PointsThe loop iterates through the list "word_1" and separates each letter into a string named "words". But strings do not have a "remove" method. Did you intend to perform that method on the list ("word_1") instead?
A few other hints:
- altering a list while iterating on it can cause items to be skipped
- you can't "join" a string, did you mean to do that on the list?
- joining with a "," will not rebuild the word, but will create a string of comma-separated letters