Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial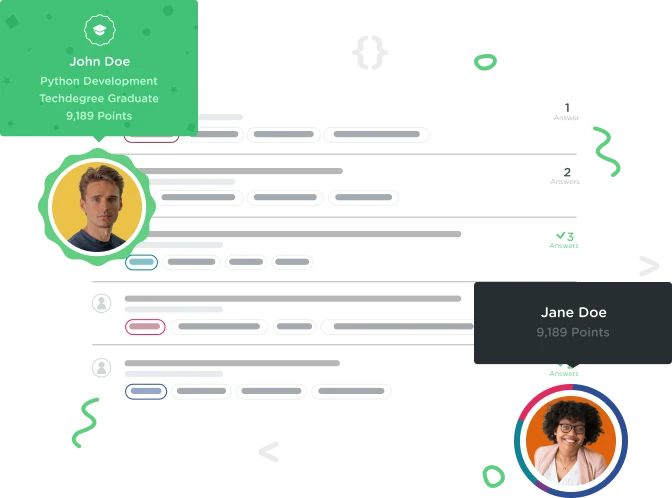

Devan Laton
602 PointsI need help on how to raise a value error
I don't know if the value error is what I did wrong, or if the "if" is wrong. It could be both but im not sure
def suggest(product_idea):
return product_idea + "inator"
if product_idea < 3
raise ValueError
1 Answer
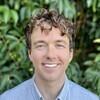
Asher Orr
Python Development Techdegree Graduate 9,409 PointsHi @Devin Laton! You're fairly close to solving the problem. I'll try to break down your code line by line.
def suggest(product_idea):
return product_idea + "inator"
The syntax for these lines of code is correct. However, this code will not pass the challenge. The first issue lies in where your return statement is located.
In most programming languages, including Python, a function takes arguments (if any), performs some operations, and returns a value. When Python encounters a return statement, it will return the value associated with it.
For example:
def suggest(product_idea):
return product_idea + "inator"
#call suggest function
suggest("Term")
#the function returns "Terminator"
Python will also exit the function after executing a return statement. It will not parse through the remaining code. Check out this example:
def suggest(product_idea):
return product_idea + "inator"
print("My name is Devan")
#call suggest function
suggest("Term")
#it still returns "Terminator"
#You will not see "My name is Devan" printed to the console...
#... since the return statement is before the print statement.
All of that to say: the return statement should be at the end of your code. Think about the logic of your function:
- check if the length of the product_idea argument is less than 3.
- If it is, raise a ValueError.
- If not, return product_idea + "inator"
Try adjusting your code to match this logical sequence.
Three more things:
Thing 1: I suggest reading this article about how to find the length of a string object in Python.
Thing 2: Remember that in Python syntax, if statements need an : at the end of them
if name == "Devan":
#print something
Thing 3: Check out how to use if statements in Python. If the length of product_idea is less than 3, you want to raise a ValueError. But if this isn't the case, you want to return product_idea + "inator". Think about how you can use if, elif, and/or else statements to achieve this.
I hope this helps. Let me know if it answers your question!