Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial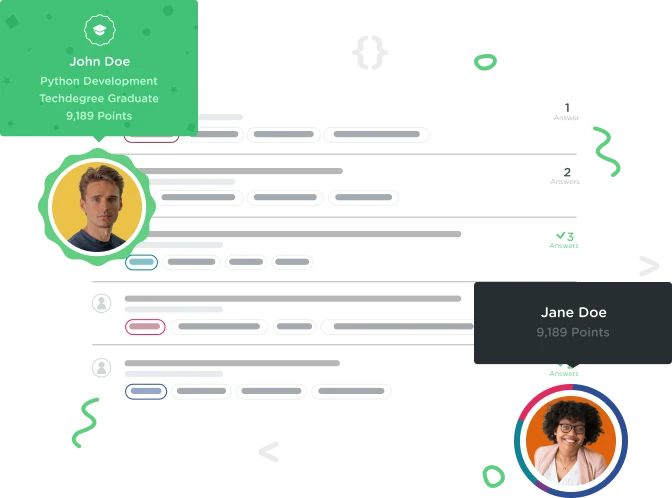

Parimal Raghavan
Courses Plus Student 1,940 PointsI need help on my game program!
Character.py:
import random
class character:
atk_range=(0,7)
def_range=(0,7)
def attack(self):
atk=random.randint(self.atk_range[0],atk_range[1])
if atk>3:
dmg=atk
return dmg
else:
return ("Attack failed!")
def dodge(self):
dodge=random.randint(self.def_range[0],def_range[1])
if dodge>3:
evd=True
return evd
else:
evd = False
return evd
Player.py:
import os
from Character import character
class player(character):
name=""
wep_inp=["s","a","b"]
def name_inp(self):
n=input("What is your name?: ")
n=n.title()
self.name=n
def wepon_inp(self):
w=input("""Choose your weapon: [S]word:6 ATK, 6 DEF
[A]xe :7 ATK, 5 DEF
[B]ow :5 ATK, 7 DEF
Enter S, A, or B :""")
if w.lower() in self.wep_inp:
if w.lower()=="s":
atk_range=(0,7)
def_range=(0,7)
elif w.lower()=="a":
atk_range=(0,8)
def_range=(0,6)
elif w.lower()=="b":
atk_range=(0,6)
def_range=(0,8)
return True
else:
return False
def __init__(self):
while True:
name_inp()
x=wepon_inp()
while x==False:
os.system("cls")
name_inp()
x=wepon_inp()
break
Game.py:
from Character import character
from Player import player
chara=player()
However, if I run Game.py, it tells me that name_inp hasn't been defined yet in Player.py! Why is that?
[MOD: fixed formatting -cf]
2 Answers
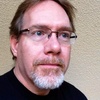
Chris Freeman
Treehouse Moderator 68,423 PointsTo refer to the methods within the Player
class code use the prefix "self" as in:
self.name_inp()
and self.wepon_inp()

Parimal Raghavan
Courses Plus Student 1,940 PointsThank you! If you don't mind, I'm probably going to run into other issues while I'm making this. Can I post my problems here, and you can help me when you get the time?
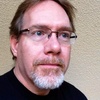
Chris Freeman
Treehouse Moderator 68,423 PointsNo worries. Post back if you have issues. It is also very appropriate to create a new post as needed.