Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial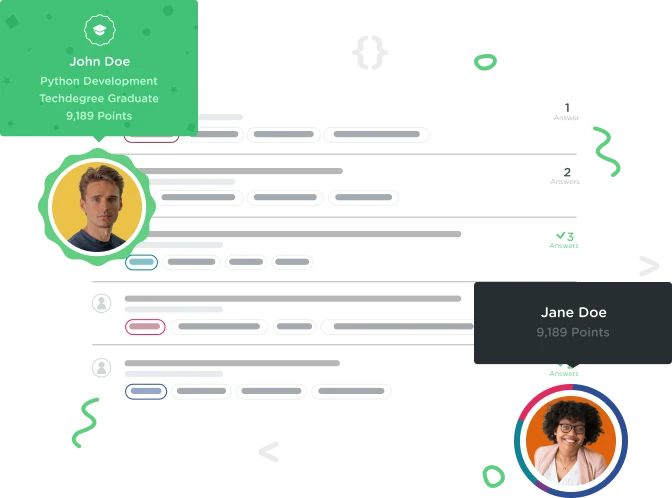
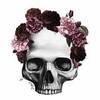
Mackenzie Milroy
3,162 PointsI need help on the code challenge in swift 3 collections and control flow with loops i'm stuck
It's asking me to compute the value of the sum inside the while loop by using the value of counter as an index value, and by retrieving values from inside the array and adding them to the sum. How would I write that? I tried retrieving the whole array and adding it and I also tried adding each integer separately and neither were correct.
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter <= numbers.count {
counter += 1
print(counter)
}
2 Answers
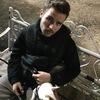
Michael Afanasiev
Courses Plus Student 15,596 PointsHi Mackenzie!
Your code is almost there! -- Let's see what the challenge is asking us;
Step 1: Create a while loop. The while loop should continue as long as the value of counter is less than the number of items in the array. (Hint: You can get that number by using the count property)
"...should continue as long as the value of counter is less than..." so we write our loop like so:
while counter < numbers {
}
"...You can get that number by using the count property..." - so we have a count property that we can use, let's add it to numbers:
while counter < numbers.count {
}
And we'll increment the counter variable not for the loop to run infinitely:
while counter < numbers.count {
counter += 1
}
Step 2: Using the value of counter as an index value, retrieve each value from the array and add it to the value of sum.
OK, so counter becomes our index value and we can use it to "call out" the numbers from the array like so:
while counter < numbers.count {
// we use counter as the index value and retrieve it from the numbers array
sum += numbers[counter]
counter += 1
}
I hope you find the step-by-step useful! ✊
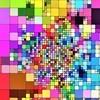
james south
Front End Web Development Techdegree Graduate 33,271 Pointsjust a few things. you don't need to print anything here. the logic is simply to use your counter as the index to the array, and progressively add each element to sum, THEN increment your counter as the last step in the loop. you can get the value at a certain index with myArray[index]. then you just need to change <= to < to get it to pass.