Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial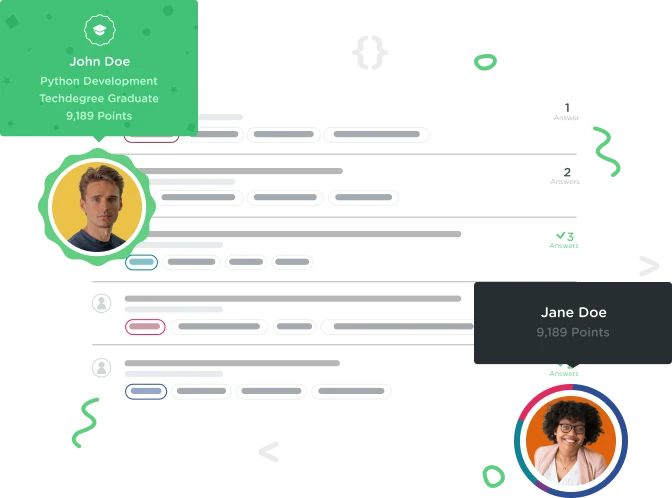
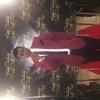
Billy Potts
Courses Plus Student 2,344 Pointsi need help overriding this method
i need to create a new class Robot which is a subclass of Machine
i need to override the method so that if you enter the string "Up" it moves up a coordinate point if you enter "Left", the x coordinate moves left
i know i can use a switch statement but im a little confused on how to go about this
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
class
4 Answers
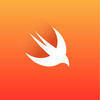
Steven Deutsch
21,046 PointsHey Billy Potts
The first thing we need to do is create your Robot class which subclasses Machine. You do this by writing a semicolon after the Robot class name and then writing the name of the Machine class.
class Robot: Machine {
}
Now inside of this class we will need to provide the override implementation for the move function. Since this method already exists in the parent class, we will need to write override before the function declaration to do this.
This method will take a single parameter, named direction, of type String. We will then switch on this direction inside the body of the method.
For the case where the direction matches the String "Up", we will increment the location.y value by 1.
For the case where the direction matches the String "Down", we will decrement the location.y value by 1.
For the case where the direction matches the String "Left", we will decrement the location.x value by 1.
For the case where the direction matches the String "Right", we will increment the location.x value by 1.
In the default case we will simply do nothing and break out of the switch statement.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Left": location.x -= 1
case "Right": location.x += 1
default: break
}
}
}
Good Luck!
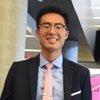
Paul Je
4,435 PointsHey @Steven,
How long did it take for you to fully get when to use dot notation, and when to use more appropriate syntax? I'm really hoping it's something that you have to practice for a really long time instead of 'getting' it earlier on haha
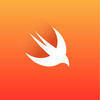
Steven Deutsch
21,046 PointsHey Paul Je,
I'm not sure how long it took me to grasp but learning to code in itself was a nightmare. There were many things that just took hours upon hours to finally click.
The thing you have to know about dot syntax is: it is the way you access the properties and methods of a type. For example:
class Square {
var width: 10
var height: 10
func area() -> Double {
return width * area
}
}
// Reason why I don't need an initializer is because all of my properties have default values
let thisShape = Square()
thisShape.width // This is how we access the width property of the Square instance
thisShape.area() // This is how we call the area method of the Square instance
Good Luck
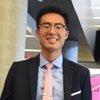
Paul Je
4,435 PointsThanks!!!
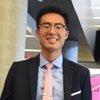
Paul Je
4,435 PointsReally good to know. Will keep plugging on
Billy Potts
Courses Plus Student 2,344 PointsBilly Potts
Courses Plus Student 2,344 Pointsthanks this really helped how you explained whats going on
Steven Deutsch
21,046 PointsSteven Deutsch
21,046 PointsNo problem. You didn't fix my typo!!!