Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial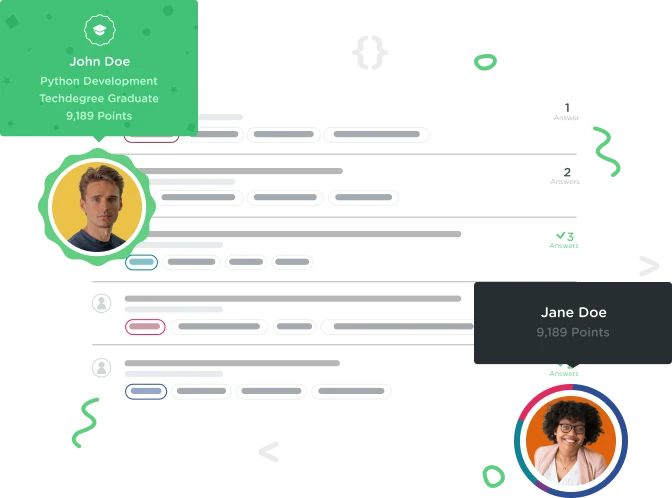
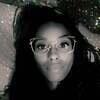
Kelinia Johnson
10,189 PointsI need help! Quickly!!! In this challenge you'll write a validator for a hexadecimal string used to set a CSS color.
I don't know how to write this string!
// Type inside this function
function isValidHex(text) {
const hexRegEx = /^(#)$ [a-f] 6 $/i.test(text);
}
const hex = document.getElementById("hex");
const body = document.getElementsByTagName("body")[0];
hex.addEventListener("input", e => {
const text = e.target.value;
const valid = isValidHex(text);
if (valid) {
body.style.backgroundColor = "rgb(176, 208, 168)";
} else {
body.style.backgroundColor = "rgb(189, 86, 86)";
}
});
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<p>Enter a valid hex value below to make the screen turn green.</p>
<input type="text" id="hex">
</div>
<script src="app.js"></script>
</body>
</html>
here's another attempt:
var hexRegEx = /"#"+[a-z]$/.test(text)
1 Answer

jb30
44,806 PointsYou have /^(#)$ [a-f] 6 $/i
. I think that the $ character should be at the end of your regular expression, before the /i, but not following the (#). You might not want a space between (#)
and [a-f]
, unless you are expecting your input to have it. If you want a value from [a-f]
to repeat 6 times, you could try [a-f]{6}
instead of having a space followed by the character 6 followed by another space followed by the end of the line.
Instead of limiting your hexadecimal numbers to using the characters a, b, c, d, e, f, or their uppercase equivalents, you might also allow them to use the digits 0, 1, 2, 3, 4, 5, 6, 7, 8, or 9, by changing [a-f]
to [\da-f]
or [a-f\d]
.
If you know that all of your colors have six hexadecimal digits, then those changes should be sufficient. However, hexadecimal colors such as #aabbcc can also be written as #abc, which your code would claim to be invalid. If you want to allow both three digit and six digit hexadecimal numbers, try /^#([\da-f]{3}){1,2}$/i
.
Kelinia Johnson
10,189 PointsKelinia Johnson
10,189 Pointsit says i need to limit my regex to meet the requirements