Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial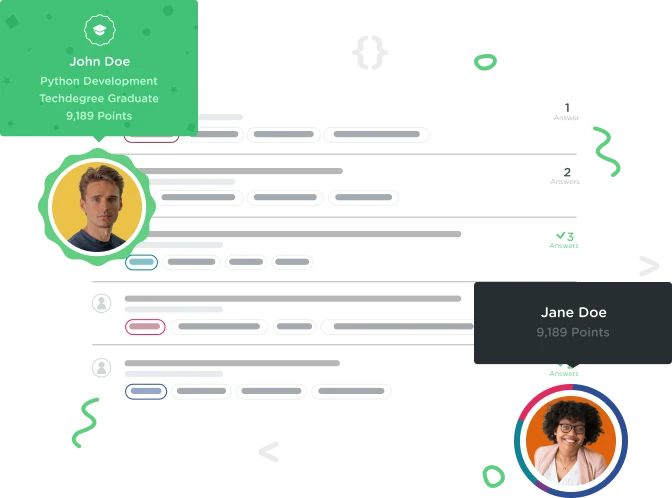

Jason Ladieu
10,635 PointsI need help to end a infinitive recursive statement in Java
I'm trying to get this method to run smoothly but I can not seem to figure out how to stop my recursive statement to infinitely. I thought I could solve it with a do..while loop but that did not work for me. Any help would be greatly appreciated!
Here is the snippet of code I'm having trouble with.
String sentence = null;
String[] conjunction = {"and", "or", "but", "because"};
int c = (int)(Math.random()*conjunction.length);
boolean repeat = false;
do {
sentence = SimpleSentence() + (conjunction[c] + Sentence());
} while (repeat == true);
return sentence;
}
Here is the full code (incase it helps to look at):
public class RandomSentences {
public static void main(String[] args) {
if (Math.random() > .5)
Sentence();
else
SimpleSentence();
}
public static String Sentence() {
String sentence = null;
String[] conjunction = {"and", "or", "but", "because"};
int c = (int)(Math.random()*conjunction.length);
boolean repeat = false;
do {
sentence = SimpleSentence() + (conjunction[c] + Sentence());
} while (repeat == true);
return sentence;
}
public static String SimpleSentence() {
String[] properNoun = {"Fred", "Jane", "Richard Nixon", "Miss America"};
String[] commonNoun = {"man", "woman", "fish", "elephant", "unicorn"};
String[] determiner = {"a", "the", "every", "some"};
String[] adjective = {"big", "tiny", "pretty", "bald"};
String[] intransitiveVerb = {"runs", "jumps", "talks", "sleeps"};
String[] transitiveVerb = {"loves", "hates", "sees", "knows", "looks for", "finds"};
int pN = (int)(Math.random()*properNoun.length);
int cN = (int)(Math.random()*commonNoun.length);
int d = (int)(Math.random()*determiner.length);
int a = (int)(Math.random()*adjective.length);
int iV = (int)(Math.random()*intransitiveVerb.length);
int tV = (int)(Math.random()*transitiveVerb.length);
String nounPhrase = null;
String verbPhrase = null;
String simpleSentence = null;
double randomNounPhrase = Math.random();
double randomVerbPhrase = (Math.random()*4) +1;
int i = (int) randomVerbPhrase;
double sentenceOrSentencePhrase = Math.random();
if (randomNounPhrase > randomNounPhrase / 2)
nounPhrase = properNoun[pN];
else
nounPhrase = determiner[d] + adjective[a] + commonNoun[cN] + "who" + verbPhrase;
switch (i) {
case 1: verbPhrase = intransitiveVerb[iV];
break;
case 2: verbPhrase = transitiveVerb[tV] + nounPhrase;
break;
case 3: verbPhrase = "is" + adjective[a];
break;
case 4: verbPhrase = "believes that" + simpleSentence;
break;
}
simpleSentence = nounPhrase + verbPhrase;
return simpleSentence;
}
}
2 Answers

Seth Kroger
56,413 PointsWith a recursive function you either return 1) a value resulting from calling the function again, or 2) return a straight value ending the recursion. The trick isn't so much figuring out how to stop but when to stop. Once you figure out determining when you should stop, the rest should be easy.
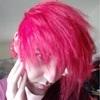
Patrik Horváth
11,110 Pointsrecursive methods stops when they see RETURN or you can throw Exception but its Dirty xD if you cant use Return so throw exception is only way to do it i think
PS use more OOP next time writing all in main method is little bit mess
for exapmle xD with additional Attribute (boolean)
public static void main(String[] args) {
boolean shouldIRepeat = true;
if (Math.random() > .5)
Sentence();
else
SimpleSentence();
}
public static String Sentence() {
String sentence = null;
String[] conjunction = {"and", "or", "but", "because"};
int c = (int)(Math.random()*conjunction.length);
boolean repeat = false;
while(shouldIRepeat) {
shouldIRepeat = false;
sentence = SimpleSentence() + (conjunction[c] + Sentence());
}
return sentence;
}
}
Patrik Horváth
11,110 PointsPatrik Horváth
11,110 Pointsrecursive methods stops when they see RETURN or you can throw Exception but its Dirty xD if you cant use Return so throw exception is only way to do it i think