Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial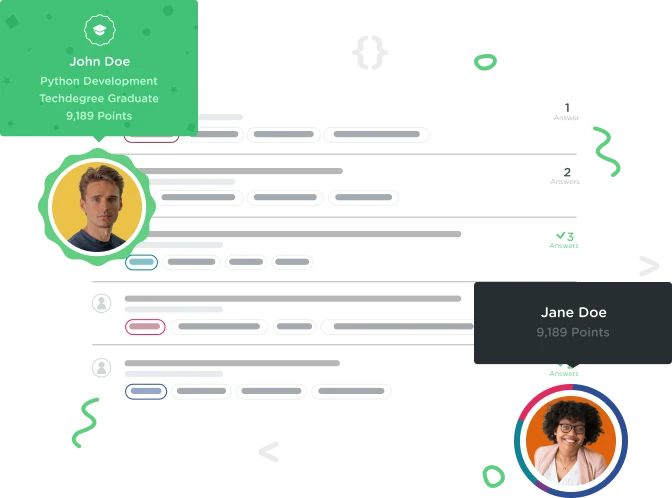

Akhilesh Bhartiya
Courses Plus Student 355 PointsI need help to replace '-' with the user entered alphabet and if its occurrence is more than one then how to deal with t
import java.util.*;
public class Hangman
{
public static void main (String[] args)
{
Scanner keyboard = new Scanner(System.in);
String wordToBeGuessed = "scratch";
String wordGuessed = "-------";
int count = 0;
while (wordGuessed != wordToBeGuessed)
{
System.out.print("Guess a letter: ");
char guessedLetter = (char) System.in.read();
//String guessedLetter = keyboard.nextLine();
int position = wordToBeGuessed.indexOf(guessedLetter);
if (position == -1)
{
System.out.println("NOT FOUND :( ! TRY AGAIN ");
}
else
{
System.out.println("Letter guessed: " +guessedLetter);
//wordGuessed.charAt(position) = guessedLetter;
//System.out.println(position);
wordGuessed.setCharAt(position, guessedLetter);
//String before = wordGuessed.substring(0,position);
//String after = wordGuessed.substring(position + 1);
//String between = guessedLetter;
//String newWord = before +between + after;
System.out.println("Display: " +wordGuessed);
}
}
}
}
letter guessed c display -c---c-
1 Answer
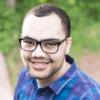
Philip Gales
15,193 PointsHere is a quick way I might do it. Notice I have intentionally left out Exceptions and other advance concepts. I also used StringBuilder in case this was your homework so your teacher would know it's not your answer. Good luck!
import java.util.Scanner;
public class Hangman {
static Scanner scanner = new Scanner(System.in);
public static void main(String[] args) {
String answer = "scratch";
StringBuilder userAnswer = new StringBuilder("-------");
String guess;
while (!userAnswer.toString().equals(answer)) {
//display blanks so user knows what they are looking for.
System.out.println(userAnswer);
//prompt user for a guess
System.out.print("Guess a letter: ");
//store user response into variable "guess"
guess = scanner.nextLine();
//convert guess to char
char c = guess.charAt(0);
//loop through each letter in answer and search for the char from user's guess
for (int i = 0; i < answer.length(); i++) {
//if the letter at position i matches the user's guess then replace the dash
if (answer.charAt(i) == c) {
userAnswer.setCharAt(i, c);
}//end if statement
}//end for loop
}//end while loop
System.out.println("Congrats, you won!");
}//end main
}
Akhilesh Bhartiya
Courses Plus Student 355 PointsAkhilesh Bhartiya
Courses Plus Student 355 Pointsthank you for your help, you forgot to put error msg if someone types wrong char and the count but i have added it into my code and it works. can i ask you one thing how do you approach a problem like this i was stuck with it for one day. trying everything but didn't work out
Philip Gales
15,193 PointsPhilip Gales
15,193 PointsI always write down ideas on paper before I start coding. I will break it down into:
Show user dashes and ask for a guess
See if guess is in answer by going through each dash
If the guess is in the answer then replace the dash with the letter
Continue asking until all dashes are replaced
I then start deciding what variables I will need (String answer, String dashes, String or char guess) and what methods or classes(maybe a method to loop through the answer and see if the guess is in the answer, if so replace the dash at the correct index)
After all of this I finally start to program, and reexamine my plan if things aren't working. It sounds like a lot, but when you have large programs, you have to write everything down and plan it all out. My old professor used to say "You eat an elephant one bite at a time".
P.S. I intentionally left out a lot of code so you would have some left to do :)