Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial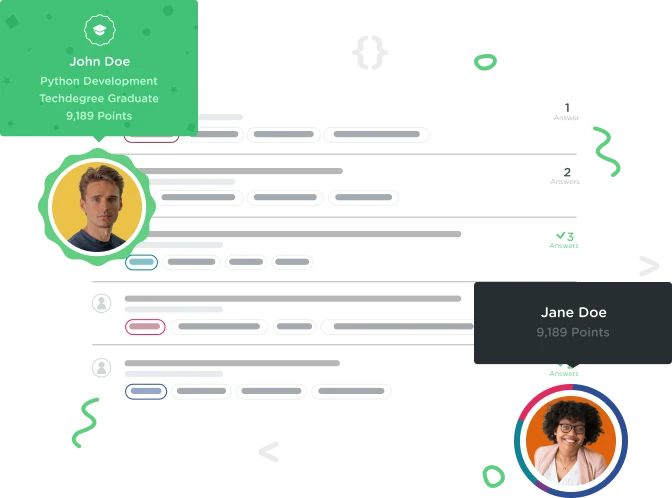

Unsubscribed User
2,446 PointsI need help understand the usage of these variables.
Like where it says
foreach(Invader invader in invaders) and FireOnInvaders(Invader[] invaders)
The usage of these variables have been confusing me constantly. Could someone please explain to me in detail how they're used? Thank you.
2 Answers
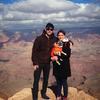
Daniel Crittenden
9,308 PointsLet's say you have a roster of football players in an array named FootballPlayers
. Each item in that array is an individual FootballPlayer
object with attributes such as their name, number, position, etc.
Let's say that you wanted to iterate through the array (AKA, the roster) FootballPlayers
and print the names of each FootballPlayer
to the console. It would probably look something like this:
foreach(FootballPlayer footballPlayer in FootballPlayers)
{
Console.WriteLine(footballPlayer.Name);
}
This would result in each football player in the array having their name printed to the console. I also want to point out something about the C# language that may be a source of confusion for you:
Pay close attention to the casing. The first FootballPlayer
indicates that this is a FootballPlayer object. Maybe in another example it's an int, double, or bool object.
The second footballPlayer
in the foreach statement above is a placeholder for the variable name. We can put almost anything in here instead. For example:
foreach(FootballPlayer **player** in FootballPlayers)
OR
foreach(FootballPlayer **x** in FootballPlayers)
OR
foreach(FootballPlayer **FutureCTECandidate** in FootballPlayers)
Note that the asterisks are added for affect and should not be included in the code.
We use that placeholder variable, whatever it is, to interact with the FootballPlayer object during the for loop....so, if the placeholder is x
, we could type x.TouchdownQuantity
to extract the number of touchdowns that player had scored.
The last FootballPlayers
(note the plural) is the variable name for the array (aka, roster) that was chosen.
Does that make sense/help at all? When all of the names are so close, I can see why it can be hard to keep up. Half the battle is understanding which aspects of the declaration are fixed and which ones you can adjust.
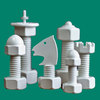
Steven Parker
230,274 PointsIn both cases "invaders" is an array of objects of the class "Invader".
In the first case, it's being used in a loop which will go through all of the items in it, one at a time.
In the other case, it's just being declared as the kind of argument that the FireOnInvaders function will take.