Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial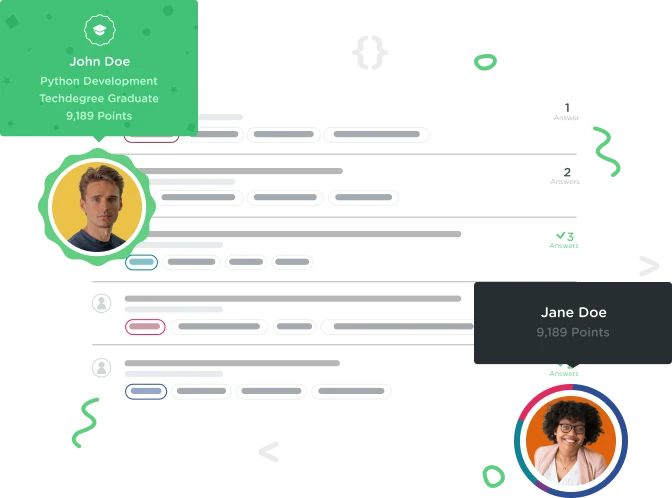

Gilbert Jaramillo Jr
725 PointsI need help with my class and we haven't gotten this far in my class with you yet
I need to get this code written. Create a Java program that takes a month number as an input and outputs the proper month name. Your program should have two classes.
The first class, Month, should include the method MonthInput, which takes a user input and stores it in a class level variable. The second method MonthName of data type String, will accept one argument, a month number, and return the proper month name (use any decision structure type in this method body). The third method, DisplayMonth, will accept a string with the name of the month found and generate a user-friendly display screen with the user input entered and the name the month found.
The second class, ShowMonth, will have the main method. It will create an object from the Month class and call each of three instance methods defined in the Month class.
How would I write it like the way you are teaching me in this class Thanks
1 Answer

Yongshuo Wang
5,500 PointsYour question is not clear, but I think the following code may what your need, hope this can help you. You can copy the code into your workspace and run the javac & java.
MonthView.java
import java.io.Console;
public class MonthView{
public static void main(String[] args){
Month m = new Month();
boolean invalid = false;
do{
Console console = System.console();
try{
String month = console.readLine("Enter month number: ");
m.setMonth(Integer.parseInt(month));
System.out.printf("Month name is %s\n", m.getMonthStr());
invalid = false;
}catch(IllegalArgumentException ex){
invalid = true;
System.out.printf("Error: %s\n", ex.getMessage());
}
}while(invalid);
}
}
Month.java
public class Month{
private int mMonth;
public Month(){
mMonth = 1;
}
public void setMonth(int month){
if (month < 1 || month > 12)
throw new IllegalArgumentException("Invalid month, month should between 1 and 12");
mMonth = month;
}
public String getMonthStr(){
String monthName = "";
switch(mMonth){
case 1:
monthName = "Jan";
break;
case 2:
monthName = "Feb";
break;
case 3:
monthName = "Mar";
break;
case 4:
monthName = "Apr";
break;
case 5:
monthName = "May";
break;
case 6:
monthName = "Jun";
break;
case 7:
monthName = "Jul";
break;
case 8:
monthName = "Aug";
break;
case 9:
monthName = "Sep";
break;
case 10:
monthName = "Oct";
break;
case 11:
monthName = "Nov";
break;
case 12:
monthName = "Dec";
break;
default:
throw new IllegalArgumentException("Invalid month.");
}
return monthName;
}
}
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherDo you have a start of the problem? Can I help you get unstuck?