Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial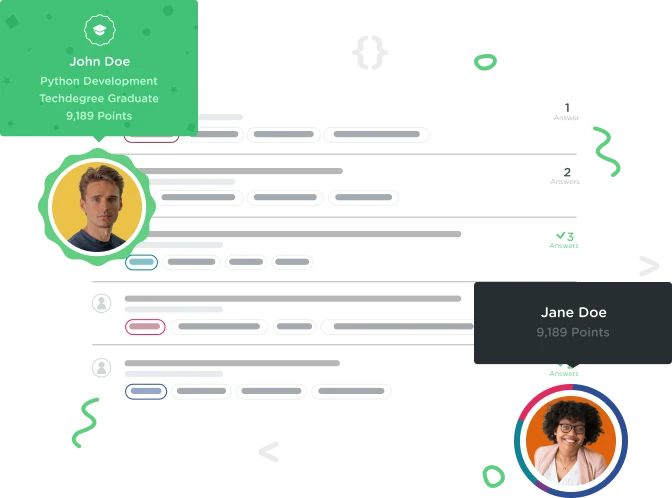

Ksenija Klimova
6,163 PointsI need Help with the Challenge Return Values in C#.
I don't understand the problem of my code, because I get the desired answer in Terminal The result I received: Bummer: We made our own separate call to Multiply, but we didn't see the result we expected for the arguments we passed! Is your Multiply method multiplying its two parameters together and calling Console.WriteLine with the result?
using System;
class Program
{
static double Multiply(double first, double second)
{
return first * second;
}
static void Main(string[] args)
{
Console. WriteLine(Multiply(2.5,2));
Console. WriteLine(Multiply(6,7));
}
}
4 Answers
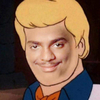
Daniel Turato
Java Web Development Techdegree Graduate 30,124 PointsThe challenge states that the result of the multiplication in the multiply method needs to be outputted from inside the method and not returned so there lies your problem. So all you have to do is this:
using System;
class Program
{
static void Multiply(double first, double second)
{
Console.WriteLine(first * second);
}
static void Main(string[] args)
{
Multiply(2.5, 2);
Multiply(6,7);
}
}

Ksenija Klimova
6,163 PointsThank you for your answer, It was really helpful:)
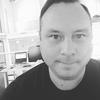
jeremiahjacquet
16,885 PointsThere seems to be an error in the test code from TeamTreehouse when verifying output using the recommended code above:
Unhandled Exception: System.NullReferenceException: Object reference not set to an instance of an object.
at Test.Main(String[] args) in /workdir/Test.cs:line 19
This post here seems to be an older challenge in C#
The current challenge is:
- Compute within the Multiply method first
- Within the Main method capture outputs in the form of variables and printout to console
- Then add the vars together
using System;
class Program
{
static double Multiply(double first, double second)
{
return (first * second);
}
static void Main(string[] args)
{
double add1 = Multiply(2.5, 2);
Console.WriteLine(add1);
double add2 = Multiply(6,7);
Console.WriteLine(add2);
Console.WriteLine(add1 + add2);
}
}
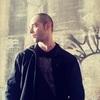
David Franco
3,252 PointsSpoiler Alert:
static double Multiply(double firstParam, double secondParam)
{
return firstParam * secondParam;
}
static void Main(string[] args)
{
double add1 = Multiply(2.5, 2);
Console.WriteLine(add1);
double add2 = Multiply(6,7);
Console.WriteLine(add2);
Console.WriteLine(add1 + add2);
}
Ksenija Klimova
6,163 PointsKsenija Klimova
6,163 PointsThank you so much for the answer! Can you please explain me the difference between output and return value?
Daniel Turato
Java Web Development Techdegree Graduate 30,124 PointsDaniel Turato
Java Web Development Techdegree Graduate 30,124 PointsOutputting a value is where you print a certain piece of data to the console, so the user/developer can see it. This is done via Console.WriteLine(). A return value is a value you specify on a method that needs to come out at the end of the process . So lets use the multiply example like above but instead with a return value:
So here in the above example, Multiply states in the method declaration that at some point in that method it needs to return a double data value. So once you call this method and it returns that value, you can then access the value returned by assigning it to another variable or passing it into another method ect. So let's say you don't want to return a value like in the challenge, in this situation you would use the void keyword.
jordansangalang
5,042 Pointsjordansangalang
5,042 PointsI have this exact code and it is still throwing an error. I copied and pasted your code here in case of any typos and I am still getting an error.
Daniel Turato
Java Web Development Techdegree Graduate 30,124 PointsDaniel Turato
Java Web Development Techdegree Graduate 30,124 PointsAre you sure you copied the right piece of code? The correct code is the one marked as best answer , not the one in the answers comments
jordansangalang
5,042 Pointsjordansangalang
5,042 PointsYes, I'm sure, because the code you pasted here was identical to my code. I restarted the quiz, pasted it again and passed on the first try. I think there was a bug of some sort. Not sure what series of events caused it though. For some reason the text editor was trying to multiply 5* 42
John San Pietro
6,870 PointsJohn San Pietro
6,870 PointsNOPE NOT CORRECT DUDE