Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial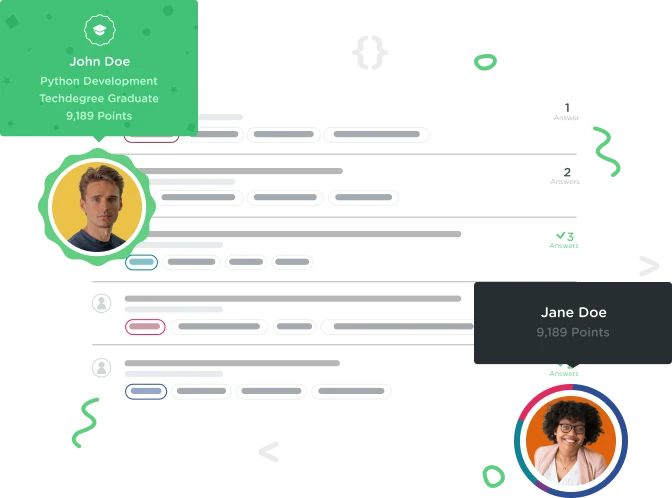

Erik L
Full Stack JavaScript Techdegree Graduate 19,470 PointsI need help with the random quote generator project
hello I am doing the Javascript tech-degree and I am working on the first project, I have a few questions :
-why do I get an error for line 55, I closed the </p> correctly? -Also if you see something else wrong in my code please let me know, below my code is shown:
// FSJS - Random Quote Generator
// Create the array of quote objects and name it quotes
var quotes = [
{ quote: 'The biggest risk is not taking any risk... In a world that changing really quickly, the only strategy that is guaranteed to fail is not taking risks.',
source: 'Mark Zuckerberg',
citation: "Facebook's Mark Zuckerberg -- Insights For Entrepreneurs",
year: '2011'
},
{
quote: 'Not everything that can be counted counts, and not everything that counts can be counted.',
source: 'William Bruce Cameron ',
citation: 'Informal Sociology: A Casual Introduction to Sociological Thinking',
year: '1963'
},
{
quote: 'Life is 10% what happens to you and 90% how you react to it.',
source: 'Charles R. Swindoll',
citation: 'https://www.brainyquote.com/quotes/charles_r_swindoll_388332',
},
{
quote: 'Our greatest weakness lies in giving up. The most certain way to succeed is always to try just one more time',
source: 'Thomas A. Edison',
citation: 'https://www.brainyquote.com/quotes/thomas_a_edison_149049',
},
{
quote: "I'd rather attempt to do something great and fail than to attempt to do nothing and succeed.",
source: 'Robert H. Schuller',
citation: 'https://www.brainyquote.com/quotes/robert_h_schuller_155998?src=t_motivational',
}
];
// Create the getRandomQuuote function and name it getRandomQuote
function getRandomQuote(array) {
var quoteIndex = Math.floor( Math.random() * (quotes.length));
for (var i = 0; i < array.length; i++) {
var randomQuote = array[quoteIndex];
}
return randomQuote;
}
var result = getRandomQuote(quotes);
console.log(result);
// Create the printQuote funtion and name it printQuote
function printQuote() {
var result = getRandomQuote();
var message = "<p class='quote'> quotes.quote + "</p>
<p class="source"> quotes.source
<span class="citation"> quotes.citation </span>
<span class="year"> quotes.year </span>
</p>
document.getElementById('quote-box').innerHTML = message;
}
// This event listener will respond to "Show another quote" button clicks
// when user clicks anywhere on the button, the "printQuote" function is called
document.getElementById('loadQuote').addEventListener("click", printQuote, false);
2 Answers
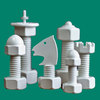
Steven Parker
231,269 PointsThe quotes are not enclosing the closing tag, but they do (and should not) enclose the variable name to be included in the string:
var message = "<p class='quote'> quotes.quote + "</p> <- error
var message = "<p class='quote'>" + result.quote + "</p>" + // fixed
The remaining lines would need to be similarly fixed to enclose the literals but not the variables, and to put concatenation operators (+) between them. Also, you can see I replaced "quotes" with "result", since the former is an array and not an object.
Alternately, you could enclose the entire message in accents ("back-ticks"), which supports both multi-line strings and interpolation of variables:
var message = `<p class="quote">${result.quote}</p>
<p class="source">${result.source}
<span class="citation">${result.citation}</span>
<span class="year">${result.year}</span>
</p>`;

Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsHey Erik L , you are doing great . And congrats on completing projects first.
But there are few issue I can see -
- you are declaring result variable twice - once as a global variable and once as a local variable.
So , you need to define it only once and this will be local to printQuote() function , because at the end printQuote is the function you will be calling withing eventListener . So , delete gloabal result variable.
-
When you are concatinating the string together you have written -
var message = "<p class='quote'> quotes.quote + "</p> <p class="source"> quotes.source <span class="citation"> quotes.citation </span> <span class="year"> quotes.year </span> </p>
which is wrong . You have to concatenate the string with variable message in each line and you also need to use semicolon at the end of each line . You have also not enclosed
</p>
within string , you have written it like you like it in html file . You must enclose it within string like this --message = "<p class='quote'>" + result.quote + "</p>"; message += "<p class='source'>" + result.source; message += "<span class='citation'>" + result.citation + "</span>"; message += "<span class='year'>" + result.year + "</span>" message += "</p>";
Don't forget to initialize the message variable with emptly string like this
var message = " "
. I also don't see any quote at when the page opened. I got the quote when I pressed the button.
So , you need to call the function printQuote once , so that , when user open the page , a quote must be there already.
So , you final code will look like this--
// Create the array of quote objects and name it quotes
var quotes = [{
quote: 'The biggest risk is not taking any risk... In a world that changing really quickly, the only strategy that is guaranteed to fail is not taking risks.',
source: 'Mark Zuckerberg',
citation: "Facebook's Mark Zuckerberg -- Insights For Entrepreneurs",
year: '2011'
},
{
quote: 'Not everything that can be counted counts, and not everything that counts can be counted.',
source: 'William Bruce Cameron ',
citation: 'Informal Sociology: A Casual Introduction to Sociological Thinking',
year: '1963'
},
{
quote: 'Life is 10% what happens to you and 90% how you react to it.',
source: 'Charles R. Swindoll',
citation: 'https://www.brainyquote.com/quotes/charles_r_swindoll_388332',
},
{
quote: 'Our greatest weakness lies in giving up. The most certain way to succeed is always to try just one more time',
source: 'Thomas A. Edison',
citation: 'https://www.brainyquote.com/quotes/thomas_a_edison_149049',
},
{
quote: "I'd rather attempt to do something great and fail than to attempt to do nothing and succeed.",
source: 'Robert H. Schuller',
citation: 'https://www.brainyquote.com/quotes/robert_h_schuller_155998?src=t_motivational',
}
];
// Create the getRandomQuuote function and name it getRandomQuote
function getRandomQuote(array) {
var quoteIndex = Math.floor(Math.random() * (quotes.length));
for (var i = 0; i < array.length; i++) {
var randomQuote = array[quoteIndex];
}
return randomQuote;
}
// Create the printQuote funtion and name it printQuote
function printQuote() {
var message = ""; // Initializing the message variable with empty string
var result = getRandomQuote(quotes);
message = "<p class='quote'>" + result.quote + "</p>";
message += "<p class='source'>" + result.source;
message += "<span class='citation'>" + result.citation + "</span>";
message += "<span class='year'>" + result.year + "</span>"
message += "</p>";
document.getElementById('quote-box').innerHTML = message;
}
printQuote(); // Calling the function atleast once , so that we don't get blank screen for the first time.
// This event listener will respond to "Show another quote" button clicks
// when user clicks anywhere on the button, the "printQuote" function is called
document.getElementById('loadQuote').addEventListener("click", printQuote, false);
Hope it will help you :)

Erik L
Full Stack JavaScript Techdegree Graduate 19,470 PointsThis helped a lot, thanks so much!!!

Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsYou are most welcome :)
Erik L
Full Stack JavaScript Techdegree Graduate 19,470 PointsErik L
Full Stack JavaScript Techdegree Graduate 19,470 PointsThanks for your input, it really helped!
Steven Parker
231,269 PointsSteven Parker
231,269 PointsErik L — Glad to help! You can mark a question solved by choosing a "best answer".
And happy coding!