Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial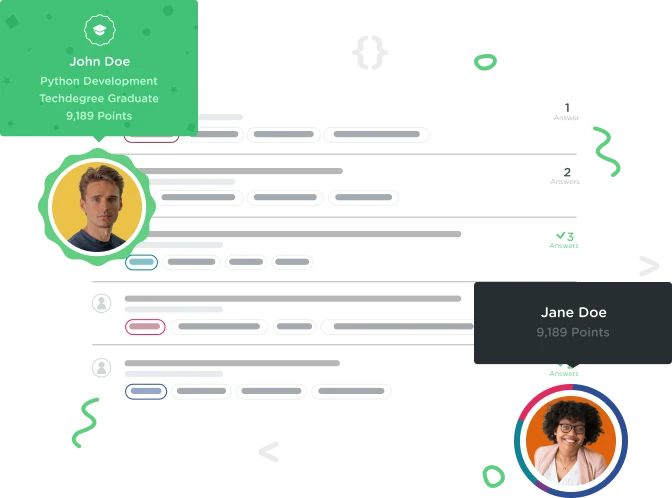
2 Answers
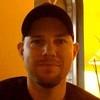
Jeremy Hill
29,567 PointsI will be happy to walk you through it:
Task 1:
it asks you to set the variable "response" equal to console.readLine("Do you understand do while loops?") In order to hold information you must declare a variable to the type of information that you are wanting to store and then you must equal it to whatever information you want it to hold; sometimes when you are working inside a method you may find yourself needing to declare a String variable (variable that holds words, phrases, etc.) but you don't quite yet have the info for it, in this case you set it to empty string, but for now, in order to complete task one we need to set it to where it will store the information that the user provides which is where the console.readLine method comes in, so with that being said your first task should look like this:
String response = console.readLine("Do you understand do while loops?")
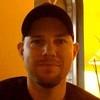
Jeremy Hill
29,567 PointsTask 2:
You must now set the variable to empty string like I mentioned before because now we have to put the readLine statement inside the do while loop; if we were to keep the readLine outside the do while loop as well as on the inside then the user would get hit first off with the the prompt for input twice. So we need to make sure that the String variable is set to empty string: "". and then you start your do while loop and inside then you just retype what you originally wrote minus the String declaration(you can only declare a variable once). After that you need to have your while condition set to tell the loop when to stop executing and finally once the condition has been met and the loop ends we need to run the code that tells the user that they passed the test. I should look like this:
String response = "";
do{
response = console.readLine("Do you understand do while loops?");
}while(response.equalsIgnoreCase("No"));
console.printf("Because you said %s, you passed the test!", response);
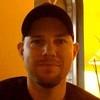
Jeremy Hill
29,567 PointsIn the while condition statement we use the built-in method equalsIgnoreCase() which will, as the title implies ignore the case of the text, which means that the user can type no with all uppercase, lowercase, or even camel case and the compiler won't care. So now the while condition statement will check the variable "response" to see if it equals something other than no, if the user keeps typing in "no" then the loop will keep looping. Once the user types in "yes" the while condition statement will see that and end the loop then it will print the String at the bottom with whatever input was received from the user.
The %s is basically a place holder for a String variable that is placed inside the quotation marks for the corresponding variable after the comma towards the end.
Let me know what doesn't make sense to you.
Jeremy Hill
29,567 PointsJeremy Hill
29,567 PointsSo the keyword "String" is the type of variable, "response" is the name that we gave it (could have been anything, with some restrictions, but to pass the challenge we have to set it to response). The keyword "console" is an object from class java.io.Console, and readLine is one of its methods. The string set inside the quotes provides info to the users so they know what to type in.