Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial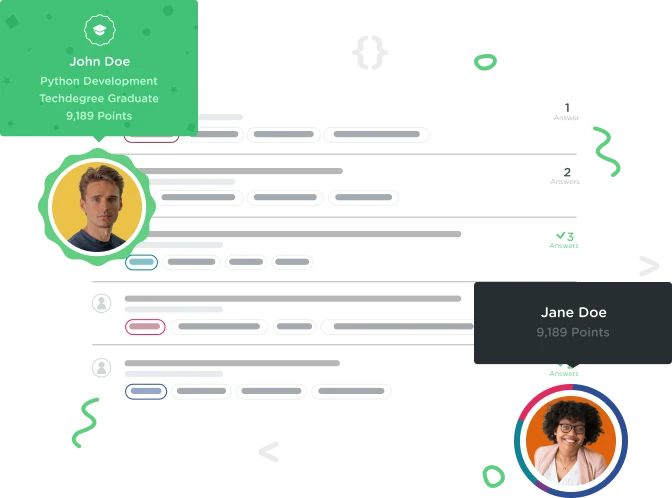

Amon Dow III
4,086 PointsI need help with this task Can someone show me the proper code and then explain it to me
I not a lot of help with them one
public class Spaceship {
public String mType;
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
public Spaceship()
{
mType= "SHUTTLE";
}
public Spaceship(String type) {
mType=type;
}
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
}
3 Answers

Ken Alger
Treehouse TeacherAmon;
Let's walk through this and hope that I don't confuse you.
Task 1
** Let's continue with our custom data model. We need some constructors. First, add a default constructor with no parameters. Inside the constructor, set mType
to "SHUTTLE".**
Prompting code:
public class Spaceship {
public String mType;
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
}
A class contains constructors that are invoked to create objects from the class blueprint. Constructor declarations look like method declarationsโexcept that they use the name of the class and have no return type. In our example, Spaceship
has one constructor that we need to make. Which we would code as public Spaceship()
. As stated that would look like a method declaration with our class name, and no return type.
The challenge then asks us to set mType
to "SHUTTLE" inside the constructor. So the entire code we need to write would be:
public Spaceship() {
mType = "SHUTTLE";
}
We would then be able to create additional spaceship objects in our code by simply writing Spaceship()
and by default it would be a type SHUTTLE.
Be sure you follow the capitalization of both the variable name and the value as both the challenge engine and Java in general are case sensitive.
I hope that helped a bit. Please post back if you are still confused.
Ken

Ken Alger
Treehouse TeacherTask 2
Now add a second constructor that takes a String parameter. Use this parameter to update mType to a specific type of spaceship.
If you are comfortable with the concepts for Task 1, Task 2 is a small step forward as it takes the same idea, but extends it to allow our program some flexibility to create Spaceships of other types. Your code for your second constructor:
public Spaceship(String type) {
mType = type;
}
... is good, you just have some additional code, at least in your post, that is not necessary. You don't need to recreate the get or setType methods.
As mentioned above, our second constructor allows us the flexibility to create a Spaceship object of additional types. How? By calling for a Spaceship("ROCKET")
object, for example. The "type" really doesn't matter as it is just a name. We could just as easily create a Spaceship("MOUSE")
object, the main point is that it allows us to create something other than a "SHUTTLE".
Hope it helps,
Ken
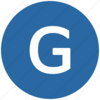
Gerardo Guzman
4,480 PointsWhat is the complete solution to....
Let's continue with our custom data model. We need some constructors. First, add a default constructor with no parameters. Inside the constructor, set mType to "SHUTTLE".