Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial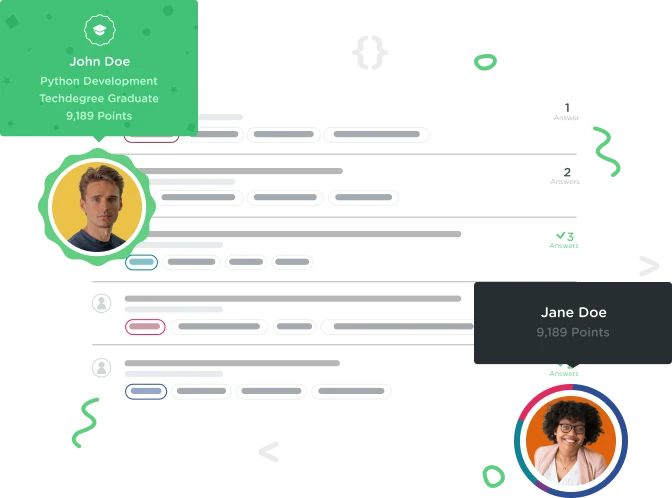
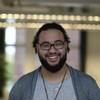
Karim Cordilia
2,380 PointsI need some help figuring out a bug
https://codepen.io/Mizukuro/pen/vMxaZZ
I have built a to do list with JavaScript, but there is one annoying bug that i can't seem to figure out.
It happens when i add multiple tasks. When you cross one off, they all get crossed off, and something weird happens when you try to edit one of the items. I've been scratching my head all day and can't seem to figure it out.
Any help would be greatly appreciated!
1 Answer
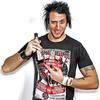
Andreas Nyström
8,887 PointsThe problem with the checkoff is that you have a eventlistener on the ul element, not the li elements. So it adds the checked class to everything inside the ul.
ul.addEventListener("click", e => {
if (e.target.tagName === "LI") {
const checked = e.target;
if (checked) {
li.classList.toggle("checked");
}
}
// localStorage["list"] = ul.innerHTML;
});
Try this instead:
li.addEventListener("click", e => {
if (e.target.tagName === "LI") {
const checked = e.target;
if (checked) {
li.classList.toggle("checked");
}
}
// localStorage["list"] = ul.innerHTML;
});
I also found that your remove didn't remove the selected item, but the first item. You can use the event and search for the closest li-element by that and then remove the child of the ul, like this:
removeBtn.addEventListener("click", (e) => {
const li = e.currentTarget.closest("li")
ul.removeChild(li);
});
And for the edit you've done kinda the same thing. Instead of using the event on the click, you're using the element itself, which will always be the first one found in the DOM. I'll try to explain..
editBtn.addEventListener("click", e => {
// Here I make a const of the closest li, which is the one you actually clicked the editbutton on.
const clickedLi = e.currentTarget.closest('li');
if (e.target.tagName == "SPAN") {
const spanEdit = e.target;
if (spanEdit.textContent === editIcon) {
// Then I use that li instead of the global li, which is always the first li, because that is the one it finds first in the DOM.
const span = clickedLi.firstElementChild;
const input = document.createElement("input");
const editBtn = clickedLi.querySelector(".edit");
input.type = "text";
input.className = "editField";
input.value = span.textContent;
clickedLi.insertBefore(input, span);
clickedLi.removeChild(span);
editBtn.textContent = saveIcon;
} else if (spanEdit.textContent === saveIcon) {
// Same thing goes for the else statement as it's the same thing happening, but in reverse.
const input = clickedLi.firstElementChild;
const span = document.createElement("span");
const editBtn = clickedLi.querySelector(".edit");
console.log(input, span, editBtn)
span.textContent = input.value;
input.textContent = span;
clickedLi.insertBefore(span, input);
clickedLi.removeChild(input);
editBtn.textContent = editIcon;
// localStorage["list"] = ul.innerHTML;
}
}
});
Alright, hope this helps. Try to do some more studying on eventListeners and how to use them. And when you're feeling happy with how your to-do-list works then try to make it functions-based. If you don't know what a function is yet, or if you're just not comfortable that would be a natural next step in your JavaScript ride :).
Hope this helps, cheers!
Karim Cordilia
2,380 PointsKarim Cordilia
2,380 PointsSuch a stupid mistake! Thank you very much for helping me solve that one. I'm curious to see what's wrong with the edit behaviour.
Thanks!
Andreas Nyström
8,887 PointsAndreas Nyström
8,887 PointsKarim Cordilia I've added more to my answer now. Hope it helps and please do study the events and eventlisteners! :)
Karim Cordilia
2,380 PointsKarim Cordilia
2,380 PointsThank you very much!