Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial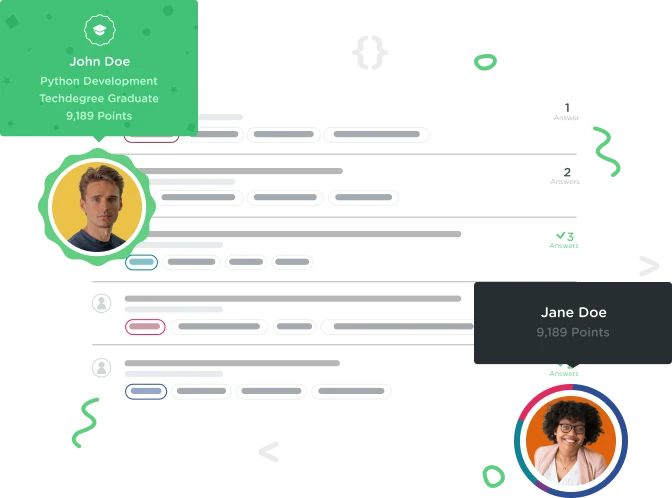

Mary Simmon
772 PointsI need some help understanding enum.
In the video(Objective C), the tutor declared an enum :
typedef enum orderItem{
Bucket = 1,
Sandwich = 2,
Soda = 3,
FamilyDeal = 4,
DoubleTrouble = 5,
LonelyBird = 6
} ordetItem;
Is the "ordetItem" misspelled? What does "ordetItem" represent when you create an enum?
1 Answer
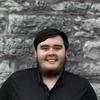
Michael Hulet
47,913 PointsYes, the instance of ordetItem
at the end appears to be misspelled and should be orderItem
like the first one. To understand what each instance of the orderItem
name means, let's break down how typedef
s and enum
s work in C
typedef
The typedef
keyword basically tells the compiler that you're gonna use a different name for a specific type. For example, if I wanted to use the keyword number
to say int
, I could write this line:
typedef int number;
Later on, if I wrote the following code, the compiler would understand that when I write number
, I really mean int
, and everything will work just fine:
number one = 1;
number two = 2;
printf("%d", one + two);
To give a type a different name like this with typedef
, you first write the type name that the compiler already knows about, then you write the name that you want to use instead
enum
You can totally declare an enum
on its own without using a typedef
. You would do so basically as you're doing in your question above, but without the typedef
syntax, like this:
enum orderItem{
Bucket = 1,
Sandwich = 2,
Soda = 3,
FamilyDeal = 4,
DoubleTrouble = 5,
LonelyBird = 6
};
However, if we wanted to declare a variable that would hold one of these values, we would still have to use the enum
keyword when we declare that variable. For example, something like this:
enum orderItem meal = FamilyDeal;
That line declares a new variable called meal
that holds one of the values of our orderItem
enumeration. Since you already know that orderItem
is an enum
, it looks a bit clunky that you have to write enum
all over again when you use one of the values, right?
Combined
In the code posted in your question, typedef
and enum
are working in concert. It's basically telling the compiler that you want an enum
called orderItem
, but instead of having to write enum orderItem
when you use one of its values, you just want to write orderItem
instead, like this:
typedef enum orderItem{
Bucket = 1,
Sandwich = 2,
Soda = 3,
FamilyDeal = 4,
DoubleTrouble = 5,
LonelyBird = 6
} orderItem;
orderItem meal = FamilyDeal;
So, going through it bit by bit, the typedef
keyword tells the compiler that you're going to refer to a type by a new name, the enum
keyword makes a new enumeration that also happens to be the old type that the compiler already knows, the first orderItem
is the compiler's name for your new enum
, then comes all the possible values, and then the 2nd orderItem
(or ordetItem
in your original question) is the name that you want to use to refer to the previously-known type (your new enum
).
The line afterwards declares a new variable called meal
that holds a value from your orderItem
enumeration using the name defined by the typedef
, and gives it a value of FamilyDeal
Mary Simmon
772 PointsMary Simmon
772 PointsThank you! You answer was very helpful!