Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial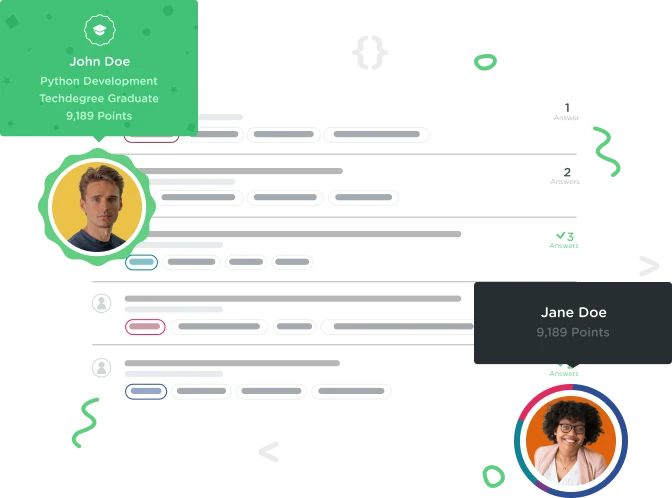
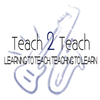
Jason Roe
1,257 PointsI need some help with this one
String [] frogNames = {
"Mike",
"J",
"Kay"};
int numberOfFrogs = frogNames.length;
String bestFrog = frogNames [numberOfFrogs];
6 Answers

Ernest Grzybowski
Treehouse Project ReviewerChallenge 1:
Declare an array of Strings named frogNames. Initialize the frogNames array with three frog names using the curly brace syntax we learned in the video. Bonus points* if one of them is Mike! (*Not really.)
You said:
String [] frogNames = {
"Mike",
"J",
"Kay"};
Correct!
Challenge 2: Declare a String variable named 'bestFrog' and initialize it to the first element of the frogNames array.
You said: (If you got to challenge 3 then you must have said)
String [] frogNames = {
"Mike",
"J",
"Kay"};
String bestFrog = frogNames [0];
Challenge 3: Declare an int variable named 'numberOfFrogs' and set it to the number of elements in the array using the array's 'length' property.
You said:
String [] frogNames = {
"Mike",
"J",
"Kay"};
int numberOfFrogs = frogNames.length;
String bestFrog = frogNames [numberOfFrogs];
This is where you go wrong.
You create the int variable correctly, but for some reason, you put it before your String bestFrog
and then you also put the numberOfFrogs
in the String initialization. This is not what the challenge is asking at all.
Since it seems like you got the right answer to the challenge, but messed up in a different part (by introducing unnecessary steps into the challenge I will post the code below for you. I hope this sets you back on track. Keep coding!
String [] frogNames = {
"Mike",
"J",
"Kay"};
String bestFrog = frogNames [0];
int numberOfFrogs = frogNames.length;

Ernest Grzybowski
Treehouse Project ReviewerWhat are you having trouble with exactly?
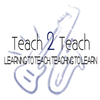
Jason Roe
1,257 PointsI keep getting the error: Bummer! Compilation Error! Double-check your syntax for creating the int variable and accessing the 'length' property of 'frogNames'.
I'm not sure I understand how to use the correct syntax.

Ernest Grzybowski
Treehouse Project ReviewerWhich challenge task exactly are you getting the error?
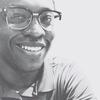
Ricardo Hill-Henry
38,442 PointsIf you're referencing the array length it will be 3. In this case there is no item in the array with an index of 3, because arrays are 0 based.

Ernest Grzybowski
Treehouse Project ReviewerThis is very true, but from what I'm seeing here... there is no challenge question that asks you to use the length of the array as the value for bestFrog.
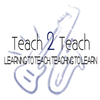
Jason Roe
1,257 PointsStage 2 challenge task 3 Declare an int variable named 'numberOfFrogs' and set it to the number of elements in the array using the array's 'length' property.
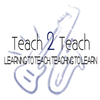
Jason Roe
1,257 PointsIs there anything wrong with this?
String[] frogNames = { "Mike", "J", "Kay"}; String bestFrog = frogNames [numberOfFrogs]; int numberOfFrogs; numberOfFrogs = frogNames.length();

Ben Jakuben
Treehouse TeacherThis is a confusing thing about arrays (and is the point of this challenge). This line is causing your error:
String bestFrog = frogNames [numberOfFrogs];
numberOfFrogs
equals 3 at this point. But the array starts with index 0. So the items in the array correspond to index 0, 1, and 2. There is no index 3. You need to adjust for the fact that the index starts at zero (just subtract one from the length to get the last index of the array).