Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial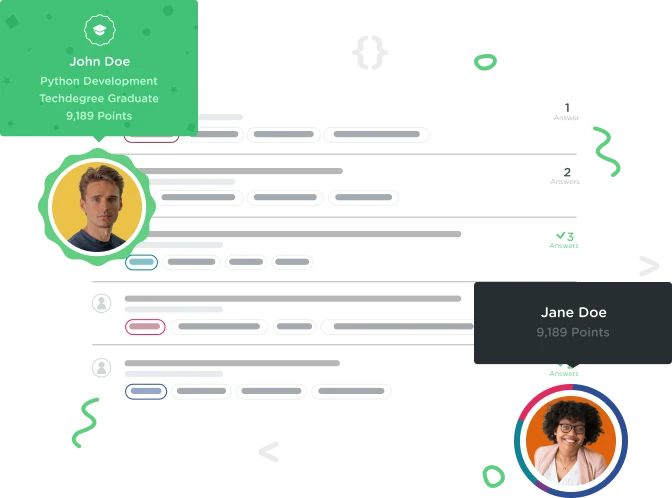

Nour El-din El-helw
8,241 PointsI need someone to review my solution to creating an owner for the pet.
So instead of creating a new Owner class, I just created the owner object inside the Pet class. I think this is a better solution, but I need someone who is more experienced than me to tell me if it is actually a better solution or not and is that good/bad practice. This is my code :
class Pet {
constructor(animal, age, breed, sound, ownerName, ownerAdress) {
this.animal = animal;
this.age = age;
this.breed = breed;
this.sound = sound;
this.owner = {
ownerName,
ownerAdress
}
}
get activity() {
const today = new Date();
const hour = today.getHours();
if (hour > 8 && hour <= 20) {
return 'playing';
} else {
return 'sleeping';
}
}
set ownerPhone(phone) {
const phoneNormalized = phone.replace(/[^0-9]/g, '');
this._phone = phoneNormalized;
}
get ownerPhone() {
this._phone;
}
speak() {
console.log(this.sound);
}
}
const ernie = new Pet('dog', 1, 'pug', 'yip yip', 'Ashley', '123 Main Adress');
const vera = new Pet('dog', 8, 'border collie', 'woof woof');
ernie.ownerPhone = '(555) 555-555';
console.log(ernie.ownerName);
console.log(ernie.ownerPhone);
1 Answer
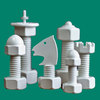
Steven Parker
231,275 PointsI think the advantage of a separate "Owner" class is that a single instance of that class might be shared by several "Pet" objects. Also, with this scheme, a pet must have an owner when it is created, but with the original structure the pet can be created before it has an owner, and the owner can easily be added or changed later.
Nour El-din El-helw
8,241 PointsNour El-din El-helw
8,241 PointsThanks a lot! :D