Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial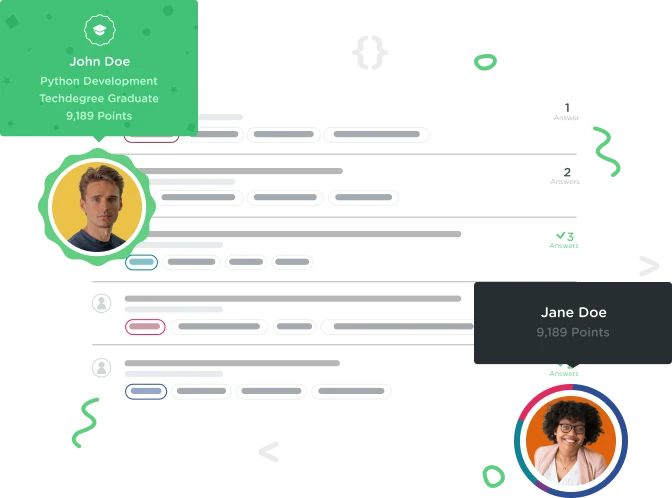

John Wetherbee
1,467 PointsI need the help of my Treehouse friends, please.
Part of my problem is understanding fully want they want. I've set up the solution, but it doesn't work. Can you help please.
Thank you,
John
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init() {
red = 86.0
green = 191.0
blue = 131.0
alpha = 1.0
}
}
let description = ("red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)")
3 Answers
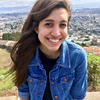
Sarah Hurtgen
Treehouse Project ReviewerSure!
The portion that needs correction is:
init() {
red = 86.0
green = 191.0
blue = 131.0
alpha = 1.0
}
}
let description = ("red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)")
They don't want you to have the 86.0, 191.0, 131.0, or 1.0 in your initializer. They were just trying to show what type of information your initializer parameters needed to be able to hold, and how your description property needed to look.
Were you able to take a look at the example in that video? Here's another example of a struct with a custom initializer:
struct Book {
let title: String
let author: String
let pageCount: Int
let bookInfo: String
// The code challenge is asking you to create a custom initializer method for your object
init(title: String, author: String, pageCount: Int) {
self.title = title
self.author = author
self.pageCount = pageCount
bookInfo = "title: \(title), author: \(author), pageCount: \(pageCount)"
}
}
They're asking you to create an initializer that allows you to input values for the first 4 variables they've listed (red, green, blue, and alpha), and then assign the String you created to the description property. For this challenge you don't need to add any code outside of the struct.
I hope that helps clear things up a little more! If you're still having a hard time getting it to accept, don't be afraid to rewatch some of the previous videos! One of the perks of treehouse is the ability to keep reviewing the info until it clicks. :)
Good luck!
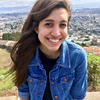
Sarah Hurtgen
Treehouse Project ReviewerHey John!
I looked it over, and they aren't wanting you to actually hardcode the example values they gave - instead they're wanting you to set up your code so that if you were to use the initializer and input that data, "description" would be structured like their example. So for this challenge all they're looking for is for you to construct the initializer.
You'll want to assign the values from the parameters (which you will create) to the stored properties they gave, using "self". If you look at the example in the Initializers and Self video about 40 seconds in, you'll see the type of structure they're looking for.
The string you have assigned to description looks great, but you'll want to move it inside the initializer and assign it to description there, so that it will be in that format every time that initializer is used.
Hope that helps!

John Wetherbee
1,467 PointsHi Sarah,
I moved my code inside the initializer, but it's not right.
Can you please provide the code I should correct.
Thank you!
John

John Wetherbee
1,467 PointsThanks very much Sarah! I certainly appreciate you helping me!!
This is what I came up with:
struct RGBColor { let red: Double let green: Double let blue: Double let alpha: Double
let description = String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double)
{
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
I tried it with and without the parentheses at the description and my response is not accepted. I went again to the forum, but if you see a minor change needed, please let me know. Thank you!
John
John Wetherbee
1,467 PointsJohn Wetherbee
1,467 PointsHi Sarah,
I hadn't added self to description.
struct RGBColor { let red: Double let green: Double let blue: Double let alpha: Double
let description: String
// Add your code below init(red: Double, green: Double, blue: Double, alpha: Double) { self.red = red self.green = green self.blue = blue self.alpha = alpha self.description = "red: (red), green: (green), blue: (blue), alpha: (alpha)" } }